mirror of
https://github.com/hyprwm/hyprlock.git
synced 2025-05-13 05:40:42 +01:00
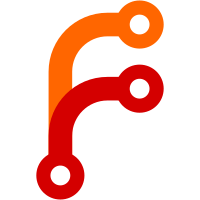
Some checks are pending
Build / nix (push) Waiting to run
* widget: add click handling and point containment methods to IWidget interface * core: add onClick method to handle mouse click events - renderer: move getOrCreateWidgetsFor method declaration to public section * core: update mouse event handling to track mouse location and button clicks * widget: add onclick command handling and point containment to CLabel - config: add onclick special config value to label * assets: add label configuration for keyboard layout switching * config: add onclick configuration for label widgets - add CLICKABLE macro for onclick configuration - replace direct onclick assignment with CLICKABLE macro * core: fix cursor shape initialization and pointer handling - ensure pointer is available before setting cursor shape - initialize cursor shape device if not already done * core: add hover handling and cursor shape updates - implement onHover method to manage widget hover states - update cursor shape based on hover status - ensure all outputs are redrawn after state changes * widgets: add hover state management and bounding box calculations - add setHover and isHovered methods to manage hover state - implement containsPoint method for hit testing - override getBoundingBox in CLabel for accurate positioning - add onHover method in CLabel to change cursor shape * core: add hover handling in pointer motion - invoke onHover method with current mouse location * widgets: add hover handling and bounding box for password input field - add getBoundingBox method to calculate the widget's bounding box - implement onHover method to update cursor shape on hover * widgets: update hover behavior for label widget - modify cursor shape setting to only apply when onclickCommand is not empty * core: optimize hover handling and rendering for lock surfaces - Improve hover state tracking for widgets - reduce unnecessary redraw calls by tracking hover changes - remove redundant renderAllOutputs() call * widgets: add onclick and hover to shape and image * core: trigger hover and onclick only for the currently focused surface * core: handle fractionalScale in onclick and hover * core: don't trigger onclick or hover when hide_cursor is set * misc: remove braces * core: run onclick commands asnychronously --------- Co-authored-by: Memoraike <memoraike@gmail.com>
73 lines
2.6 KiB
C++
73 lines
2.6 KiB
C++
#pragma once
|
|
|
|
#include <chrono>
|
|
#include <optional>
|
|
#include "Shader.hpp"
|
|
#include "../defines.hpp"
|
|
#include "../core/LockSurface.hpp"
|
|
#include "../helpers/AnimatedVariable.hpp"
|
|
#include "../helpers/Color.hpp"
|
|
#include "AsyncResourceGatherer.hpp"
|
|
#include "../config/ConfigDataValues.hpp"
|
|
#include "widgets/IWidget.hpp"
|
|
#include "Framebuffer.hpp"
|
|
|
|
typedef std::unordered_map<OUTPUTID, std::vector<SP<IWidget>>> widgetMap_t;
|
|
|
|
class CRenderer {
|
|
public:
|
|
CRenderer();
|
|
|
|
struct SRenderFeedback {
|
|
bool needsFrame = false;
|
|
};
|
|
|
|
struct SBlurParams {
|
|
int size = 0, passes = 0;
|
|
float noise = 0, contrast = 0, brightness = 0, vibrancy = 0, vibrancy_darkness = 0;
|
|
std::optional<CHyprColor> colorize;
|
|
float boostA = 1.0;
|
|
};
|
|
|
|
SRenderFeedback renderLock(const CSessionLockSurface& surf);
|
|
|
|
void renderRect(const CBox& box, const CHyprColor& col, int rounding = 0);
|
|
void renderBorder(const CBox& box, const CGradientValueData& gradient, int thickness, int rounding = 0, float alpha = 1.0);
|
|
void renderTexture(const CBox& box, const CTexture& tex, float a = 1.0, int rounding = 0, std::optional<eTransform> tr = {});
|
|
void renderTextureMix(const CBox& box, const CTexture& tex, const CTexture& tex2, float a = 1.0, float mixFactor = 0.0, int rounding = 0, std::optional<eTransform> tr = {});
|
|
void blurFB(const CFramebuffer& outfb, SBlurParams params);
|
|
|
|
UP<CAsyncResourceGatherer> asyncResourceGatherer;
|
|
std::chrono::system_clock::time_point firstFullFrameTime;
|
|
|
|
void pushFb(GLint fb);
|
|
void popFb();
|
|
|
|
void removeWidgetsFor(OUTPUTID id);
|
|
void reconfigureWidgetsFor(OUTPUTID id);
|
|
|
|
void startFadeIn();
|
|
void startFadeOut(bool unlock = false, bool immediate = true);
|
|
std::vector<SP<IWidget>>& getOrCreateWidgetsFor(const CSessionLockSurface& surf);
|
|
|
|
private:
|
|
widgetMap_t widgets;
|
|
|
|
CShader rectShader;
|
|
CShader texShader;
|
|
CShader texMixShader;
|
|
CShader blurShader1;
|
|
CShader blurShader2;
|
|
CShader blurPrepareShader;
|
|
CShader blurFinishShader;
|
|
CShader borderShader;
|
|
|
|
Mat3x3 projMatrix = Mat3x3::identity();
|
|
Mat3x3 projection;
|
|
|
|
PHLANIMVAR<float> opacity;
|
|
|
|
std::vector<GLint> boundFBs;
|
|
};
|
|
|
|
inline UP<CRenderer> g_pRenderer;
|