mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
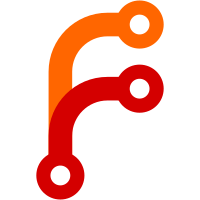
This is a reland of commit b46c4bf27b
Original change's description:
> [ACM] iSAC audio codec removed
>
> Note: this CL has to leave behind one part of iSAC, which is its VAD
> currently used by AGC1 in APM. The target visibility has been
> restricted and the VAD will be removed together with AGC1 when the
> time comes.
>
> Tested: see https://chromium-review.googlesource.com/c/chromium/src/+/4013319
>
> Bug: webrtc:14450
> Change-Id: I69cc518b16280eae62a1f1977cdbfa24c08cf5f9
> Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/282421
> Reviewed-by: Henrik Lundin <henrik.lundin@webrtc.org>
> Reviewed-by: Sam Zackrisson <saza@webrtc.org>
> Reviewed-by: Henrik Boström <hbos@webrtc.org>
> Commit-Queue: Alessio Bazzica <alessiob@webrtc.org>
> Cr-Commit-Position: refs/heads/main@{#38652}
Bug: webrtc:14450
Change-Id: Ia22c4d7724b6022238235fede93e36e570a49376
Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/283843
Reviewed-by: Henrik Lundin <henrik.lundin@webrtc.org>
Commit-Queue: Alessio Bazzica <alessiob@webrtc.org>
Reviewed-by: Sam Zackrisson <saza@webrtc.org>
Reviewed-by: Henrik Boström <hbos@webrtc.org>
Cr-Commit-Position: refs/heads/main@{#38665}
74 lines
2.3 KiB
C++
74 lines
2.3 KiB
C++
/*
|
|
* Copyright (c) 2017 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "api/audio_codecs/builtin_audio_encoder_factory.h"
|
|
|
|
#include <memory>
|
|
#include <vector>
|
|
|
|
#include "api/audio_codecs/L16/audio_encoder_L16.h"
|
|
#include "api/audio_codecs/audio_encoder_factory_template.h"
|
|
#include "api/audio_codecs/g711/audio_encoder_g711.h"
|
|
#include "api/audio_codecs/g722/audio_encoder_g722.h"
|
|
#if WEBRTC_USE_BUILTIN_ILBC
|
|
#include "api/audio_codecs/ilbc/audio_encoder_ilbc.h" // nogncheck
|
|
#endif
|
|
#if WEBRTC_USE_BUILTIN_OPUS
|
|
#include "api/audio_codecs/opus/audio_encoder_multi_channel_opus.h"
|
|
#include "api/audio_codecs/opus/audio_encoder_opus.h" // nogncheck
|
|
#endif
|
|
|
|
namespace webrtc {
|
|
|
|
namespace {
|
|
|
|
// Modify an audio encoder to not advertise support for anything.
|
|
template <typename T>
|
|
struct NotAdvertised {
|
|
using Config = typename T::Config;
|
|
static absl::optional<Config> SdpToConfig(
|
|
const SdpAudioFormat& audio_format) {
|
|
return T::SdpToConfig(audio_format);
|
|
}
|
|
static void AppendSupportedEncoders(std::vector<AudioCodecSpec>* specs) {
|
|
// Don't advertise support for anything.
|
|
}
|
|
static AudioCodecInfo QueryAudioEncoder(const Config& config) {
|
|
return T::QueryAudioEncoder(config);
|
|
}
|
|
static std::unique_ptr<AudioEncoder> MakeAudioEncoder(
|
|
const Config& config,
|
|
int payload_type,
|
|
absl::optional<AudioCodecPairId> codec_pair_id = absl::nullopt,
|
|
const FieldTrialsView* field_trials = nullptr) {
|
|
return T::MakeAudioEncoder(config, payload_type, codec_pair_id,
|
|
field_trials);
|
|
}
|
|
};
|
|
|
|
} // namespace
|
|
|
|
rtc::scoped_refptr<AudioEncoderFactory> CreateBuiltinAudioEncoderFactory() {
|
|
return CreateAudioEncoderFactory<
|
|
|
|
#if WEBRTC_USE_BUILTIN_OPUS
|
|
AudioEncoderOpus, NotAdvertised<AudioEncoderMultiChannelOpus>,
|
|
#endif
|
|
|
|
AudioEncoderG722,
|
|
|
|
#if WEBRTC_USE_BUILTIN_ILBC
|
|
AudioEncoderIlbc,
|
|
#endif
|
|
|
|
AudioEncoderG711, NotAdvertised<AudioEncoderL16>>();
|
|
}
|
|
|
|
} // namespace webrtc
|