mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
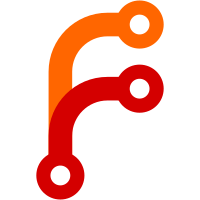
This CL decouples NetEqFactory and AudioDecoderFactory. AudioDecoderFactory is used in more places than just inside of NetEq, so decoupling these makes sense. Bug: webrtc:11005 Change-Id: I78dd856e4248e398e69a65816b062ef30555b055 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/161005 Reviewed-by: Karl Wiberg <kwiberg@webrtc.org> Commit-Queue: Ivo Creusen <ivoc@webrtc.org> Cr-Commit-Position: refs/heads/master@{#29961}
44 lines
1.5 KiB
C++
44 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2019 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef API_NETEQ_CUSTOM_NETEQ_FACTORY_H_
|
|
#define API_NETEQ_CUSTOM_NETEQ_FACTORY_H_
|
|
|
|
#include <memory>
|
|
|
|
#include "api/audio_codecs/audio_decoder_factory.h"
|
|
#include "api/neteq/neteq_controller_factory.h"
|
|
#include "api/neteq/neteq_factory.h"
|
|
#include "api/scoped_refptr.h"
|
|
#include "system_wrappers/include/clock.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// This factory can be used to generate NetEq instances that make use of a
|
|
// custom NetEqControllerFactory.
|
|
class CustomNetEqFactory : public NetEqFactory {
|
|
public:
|
|
explicit CustomNetEqFactory(
|
|
std::unique_ptr<NetEqControllerFactory> controller_factory);
|
|
~CustomNetEqFactory() override;
|
|
CustomNetEqFactory(const CustomNetEqFactory&) = delete;
|
|
CustomNetEqFactory& operator=(const CustomNetEqFactory&) = delete;
|
|
|
|
std::unique_ptr<NetEq> CreateNetEq(
|
|
const NetEq::Config& config,
|
|
const rtc::scoped_refptr<AudioDecoderFactory>& decoder_factory,
|
|
Clock* clock) const override;
|
|
|
|
private:
|
|
std::unique_ptr<NetEqControllerFactory> controller_factory_;
|
|
};
|
|
|
|
} // namespace webrtc
|
|
#endif // API_NETEQ_CUSTOM_NETEQ_FACTORY_H_
|