mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
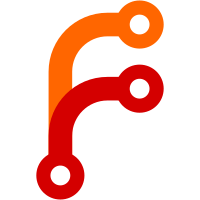
This reverts commit 8039cdbe48
.
Reason for revert: remove functionality after measurement complete
Original change's description:
> Measure wall clock time of capture and encode processing.
>
> (NOTE: This and dependent CLs will be reverted in a few days after
> data collection from the field is complete.)
>
> This change introduces a new task queue concept, Voucher. They
> are associated with a currently running task tree. Whenever
> tasks are posted, the current voucher is inherited and set as
> current in the new task.
>
> The voucher exists for as long as there are direct and indirect
> tasks running that descend from the task where the voucher was
> created.
>
> Vouchers aggregate application-specific attachments, which perform
> logic unrelated to Voucher progression. This particular change adds
> an attachment that measures time from capture to all encode operations
> complete, and places it into the WebRTC.Video.CaptureToSendTimeMs UMA.
>
> An accompanying Chrome change crrev.com/c/4992282 ensures survival of
> vouchers across certain Mojo IPC.
>
> Bug: chromium:1498378
> Change-Id: I2a27800a4e5504f219d8b9d33c56a48904cf6dde
> Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/325400
> Reviewed-by: Harald Alvestrand <hta@webrtc.org>
> Commit-Queue: Markus Handell <handellm@webrtc.org>
> Cr-Commit-Position: refs/heads/main@{#41061}
Bug: chromium:1498378
Change-Id: I9503575fbc52f1946ca26fc3c17b623ea75cd3c5
Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/327023
Commit-Queue: Markus Handell <handellm@webrtc.org>
Reviewed-by: Harald Alvestrand <hta@webrtc.org>
Bot-Commit: rubber-stamper@appspot.gserviceaccount.com <rubber-stamper@appspot.gserviceaccount.com>
Cr-Commit-Position: refs/heads/main@{#41135}
81 lines
2 KiB
C++
81 lines
2 KiB
C++
/*
|
|
* Copyright 2019 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#include "api/task_queue/task_queue_base.h"
|
|
|
|
#include "absl/base/attributes.h"
|
|
#include "absl/base/config.h"
|
|
#include "absl/functional/any_invocable.h"
|
|
#include "api/units/time_delta.h"
|
|
#include "rtc_base/checks.h"
|
|
|
|
#if defined(ABSL_HAVE_THREAD_LOCAL)
|
|
|
|
namespace webrtc {
|
|
namespace {
|
|
|
|
ABSL_CONST_INIT thread_local TaskQueueBase* current = nullptr;
|
|
|
|
} // namespace
|
|
|
|
TaskQueueBase* TaskQueueBase::Current() {
|
|
return current;
|
|
}
|
|
|
|
TaskQueueBase::CurrentTaskQueueSetter::CurrentTaskQueueSetter(
|
|
TaskQueueBase* task_queue)
|
|
: previous_(current) {
|
|
current = task_queue;
|
|
}
|
|
|
|
TaskQueueBase::CurrentTaskQueueSetter::~CurrentTaskQueueSetter() {
|
|
current = previous_;
|
|
}
|
|
} // namespace webrtc
|
|
|
|
#elif defined(WEBRTC_POSIX)
|
|
|
|
#include <pthread.h>
|
|
|
|
namespace webrtc {
|
|
namespace {
|
|
|
|
ABSL_CONST_INIT pthread_key_t g_queue_ptr_tls = 0;
|
|
|
|
void InitializeTls() {
|
|
RTC_CHECK(pthread_key_create(&g_queue_ptr_tls, nullptr) == 0);
|
|
}
|
|
|
|
pthread_key_t GetQueuePtrTls() {
|
|
static pthread_once_t init_once = PTHREAD_ONCE_INIT;
|
|
RTC_CHECK(pthread_once(&init_once, &InitializeTls) == 0);
|
|
return g_queue_ptr_tls;
|
|
}
|
|
|
|
} // namespace
|
|
|
|
TaskQueueBase* TaskQueueBase::Current() {
|
|
return static_cast<TaskQueueBase*>(pthread_getspecific(GetQueuePtrTls()));
|
|
}
|
|
|
|
TaskQueueBase::CurrentTaskQueueSetter::CurrentTaskQueueSetter(
|
|
TaskQueueBase* task_queue)
|
|
: previous_(TaskQueueBase::Current()) {
|
|
pthread_setspecific(GetQueuePtrTls(), task_queue);
|
|
}
|
|
|
|
TaskQueueBase::CurrentTaskQueueSetter::~CurrentTaskQueueSetter() {
|
|
pthread_setspecific(GetQueuePtrTls(), previous_);
|
|
}
|
|
|
|
} // namespace webrtc
|
|
|
|
#else
|
|
#error Unsupported platform
|
|
#endif
|