mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
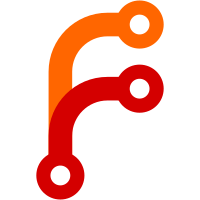
which allows determining what codec (data format) is used. Chromium CL: https://chromium-review.googlesource.com/c/chromium/src/+/4941907 Split from https://webrtc-review.googlesource.com/c/src/+/318283 to reduce CL size and avoid audio woes. BUG=webrtc:15579 Change-Id: I404107af526df3009c16d2a6148784fe87dfa807 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/323721 Reviewed-by: Tony Herre <herre@google.com> Reviewed-by: Harald Alvestrand <hta@webrtc.org> Commit-Queue: Philipp Hancke <phancke@microsoft.com> Cr-Commit-Position: refs/heads/main@{#41007}
52 lines
1.8 KiB
C++
52 lines
1.8 KiB
C++
/*
|
|
* Copyright (c) 2020 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef API_TEST_MOCK_TRANSFORMABLE_VIDEO_FRAME_H_
|
|
#define API_TEST_MOCK_TRANSFORMABLE_VIDEO_FRAME_H_
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "api/frame_transformer_interface.h"
|
|
#include "test/gmock.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class MockTransformableVideoFrame
|
|
: public webrtc::TransformableVideoFrameInterface {
|
|
public:
|
|
MOCK_METHOD(rtc::ArrayView<const uint8_t>, GetData, (), (const, override));
|
|
MOCK_METHOD(void, SetData, (rtc::ArrayView<const uint8_t> data), (override));
|
|
MOCK_METHOD(uint32_t, GetTimestamp, (), (const, override));
|
|
MOCK_METHOD(void, SetRTPTimestamp, (uint32_t), (override));
|
|
MOCK_METHOD(uint32_t, GetSsrc, (), (const, override));
|
|
MOCK_METHOD(bool, IsKeyFrame, (), (const, override));
|
|
MOCK_METHOD(void,
|
|
SetMetadata,
|
|
(const webrtc::VideoFrameMetadata&),
|
|
(override));
|
|
MOCK_METHOD(uint8_t, GetPayloadType, (), (const, override));
|
|
MOCK_METHOD(TransformableFrameInterface::Direction,
|
|
GetDirection,
|
|
(),
|
|
(const, override));
|
|
MOCK_METHOD(std::string, GetMimeType, (), (const, override));
|
|
MOCK_METHOD(VideoFrameMetadata, Metadata, (), (const, override));
|
|
MOCK_METHOD(absl::optional<Timestamp>,
|
|
GetCaptureTimeIdentifier,
|
|
(),
|
|
(const, override));
|
|
};
|
|
|
|
static_assert(!std::is_abstract_v<MockTransformableVideoFrame>, "");
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // API_TEST_MOCK_TRANSFORMABLE_VIDEO_FRAME_H_
|