mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
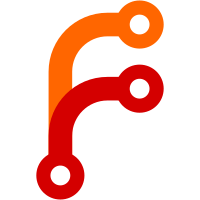
This CL introduces the Adaptation class used by VideoStreamRestrictor. This refactors the AdaptationTarget, AdaptationTargetOrReason, CannotAdaptReason and AdaptationAction. What is publicly exposed is simply a Status code. If it's kValid then we can adapt, otherwise the status code describes why we can't adapt (just like CannotAdaptReason prior to this CL). This means AdaptationTargetOrReason is no longer needed. Target+reason are merged. The other classes are renamed and moved and put in the private namespace of Adaptation: Only the VideoStreamAdapter (now a friend class of Adaptation) and its inner class VideoSourceRestrictor needs to know how to execute the adaptation. Publicly, you can now tell the effects of the adaptation without applying it with PeekNextRestrictions() - both current and next steps are described in terms of VideoSourceRestrictions. The rest are hidden. This would make it possible, in the future, for a Resource to accept or reject a proposed Adaptation by examining the resulting frame rate and resolution described by the resulting restrictions. E.g. even if we are not overusing bandwidth at the moment, the BW resource can prevent us from applying a restriction that would exceed the BW limit before we apply it. This CL also moves input to a SetInput() method, and Increase/Decrease methods of VideoSourceRestrictor are made private in favor of ApplyAdaptationSteps(). Bug: webrtc:11393 Change-Id: Ie5e2181836ab3713b8021c1a152694ca745aeb0d Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/170111 Commit-Queue: Henrik Boström <hbos@webrtc.org> Reviewed-by: Ilya Nikolaevskiy <ilnik@webrtc.org> Reviewed-by: Evan Shrubsole <eshr@google.com> Cr-Commit-Position: refs/heads/master@{#30794}
54 lines
1.7 KiB
C++
54 lines
1.7 KiB
C++
/*
|
|
* Copyright 2020 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "call/adaptation/encoder_settings.h"
|
|
|
|
#include <utility>
|
|
|
|
namespace webrtc {
|
|
|
|
EncoderSettings::EncoderSettings(VideoEncoder::EncoderInfo encoder_info,
|
|
VideoEncoderConfig encoder_config,
|
|
VideoCodec video_codec)
|
|
: encoder_info_(std::move(encoder_info)),
|
|
encoder_config_(std::move(encoder_config)),
|
|
video_codec_(std::move(video_codec)) {}
|
|
|
|
EncoderSettings::EncoderSettings(const EncoderSettings& other)
|
|
: encoder_info_(other.encoder_info_),
|
|
encoder_config_(other.encoder_config_.Copy()),
|
|
video_codec_(other.video_codec_) {}
|
|
|
|
EncoderSettings& EncoderSettings::operator=(const EncoderSettings& other) {
|
|
encoder_info_ = other.encoder_info_;
|
|
encoder_config_ = other.encoder_config_.Copy();
|
|
video_codec_ = other.video_codec_;
|
|
return *this;
|
|
}
|
|
|
|
const VideoEncoder::EncoderInfo& EncoderSettings::encoder_info() const {
|
|
return encoder_info_;
|
|
}
|
|
|
|
const VideoEncoderConfig& EncoderSettings::encoder_config() const {
|
|
return encoder_config_;
|
|
}
|
|
|
|
const VideoCodec& EncoderSettings::video_codec() const {
|
|
return video_codec_;
|
|
}
|
|
|
|
VideoCodecType GetVideoCodecTypeOrGeneric(
|
|
const absl::optional<EncoderSettings>& settings) {
|
|
return settings.has_value() ? settings->encoder_config().codec_type
|
|
: kVideoCodecGeneric;
|
|
}
|
|
|
|
} // namespace webrtc
|