mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
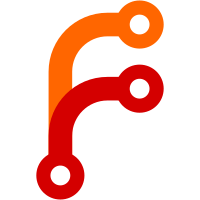
Rename RtpVideoSender::SetActiveModules to SetSending to better match what it does. When a RtpVideoSender::SetSending(true) RTP packets can be sent on all associcated RTP streams (simulcast streams). Move registration of RtpRtcpModules to RtpTransportControllerSend to allow RtpTransportControllerSend to know when there are sending RTP streams. Purpose is to in later CLs allow RtpTransportControllerSend to trigger BWE probes. Bug: webrtc:14928 Change-Id: Ibf6c040b86713cdc4763c4691b7fd794b251eb49 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/335961 Reviewed-by: Erik Språng <sprang@webrtc.org> Commit-Queue: Per Kjellander <perkj@webrtc.org> Cr-Commit-Position: refs/heads/main@{#41620}
65 lines
2.5 KiB
C++
65 lines
2.5 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef CALL_RTP_VIDEO_SENDER_INTERFACE_H_
|
|
#define CALL_RTP_VIDEO_SENDER_INTERFACE_H_
|
|
|
|
#include <map>
|
|
#include <vector>
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "api/array_view.h"
|
|
#include "api/call/bitrate_allocation.h"
|
|
#include "api/fec_controller_override.h"
|
|
#include "api/video/video_layers_allocation.h"
|
|
#include "call/rtp_config.h"
|
|
#include "modules/rtp_rtcp/include/rtp_rtcp_defines.h"
|
|
#include "modules/rtp_rtcp/source/rtp_sequence_number_map.h"
|
|
#include "modules/video_coding/include/video_codec_interface.h"
|
|
|
|
namespace webrtc {
|
|
class VideoBitrateAllocation;
|
|
struct FecProtectionParams;
|
|
|
|
class RtpVideoSenderInterface : public EncodedImageCallback,
|
|
public FecControllerOverride {
|
|
public:
|
|
// Sets weather or not RTP packets is allowed to be sent on this sender.
|
|
virtual void SetSending(bool enabled) = 0;
|
|
virtual bool IsActive() = 0;
|
|
|
|
virtual void OnNetworkAvailability(bool network_available) = 0;
|
|
virtual std::map<uint32_t, RtpState> GetRtpStates() const = 0;
|
|
virtual std::map<uint32_t, RtpPayloadState> GetRtpPayloadStates() const = 0;
|
|
|
|
virtual void DeliverRtcp(const uint8_t* packet, size_t length) = 0;
|
|
|
|
virtual void OnBitrateAllocationUpdated(
|
|
const VideoBitrateAllocation& bitrate) = 0;
|
|
virtual void OnVideoLayersAllocationUpdated(
|
|
const VideoLayersAllocation& allocation) = 0;
|
|
virtual void OnBitrateUpdated(BitrateAllocationUpdate update,
|
|
int framerate) = 0;
|
|
virtual void OnTransportOverheadChanged(
|
|
size_t transport_overhead_bytes_per_packet) = 0;
|
|
virtual uint32_t GetPayloadBitrateBps() const = 0;
|
|
virtual uint32_t GetProtectionBitrateBps() const = 0;
|
|
virtual void SetEncodingData(size_t width,
|
|
size_t height,
|
|
size_t num_temporal_layers) = 0;
|
|
virtual std::vector<RtpSequenceNumberMap::Info> GetSentRtpPacketInfos(
|
|
uint32_t ssrc,
|
|
rtc::ArrayView<const uint16_t> sequence_numbers) const = 0;
|
|
|
|
// Implements FecControllerOverride.
|
|
void SetFecAllowed(bool fec_allowed) override = 0;
|
|
};
|
|
} // namespace webrtc
|
|
#endif // CALL_RTP_VIDEO_SENDER_INTERFACE_H_
|