mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
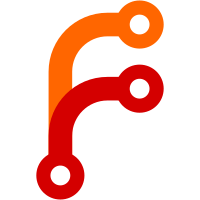
Currently there's an implicit requirement that users of SimulatedNetwork should call it repeatedly, even if the return value of NextDeliveryTimeUs is unset. With this change, it will indicate that there might be a delivery in 5 ms at any time there are packets in queue. Which results in unchanged behavior compared to current usage but allows new users to expect robust behavior. Bug: webrtc:9510 Change-Id: I45b8b5f1f0d3d13a8ec9b163d4011c5f01a53069 Reviewed-on: https://webrtc-review.googlesource.com/c/120402 Commit-Queue: Sebastian Jansson <srte@webrtc.org> Reviewed-by: Christoffer Rodbro <crodbro@webrtc.org> Cr-Commit-Position: refs/heads/master@{#26617}
42 lines
1.5 KiB
C++
42 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef CALL_SIMULATED_PACKET_RECEIVER_H_
|
|
#define CALL_SIMULATED_PACKET_RECEIVER_H_
|
|
|
|
#include "api/test/simulated_network.h"
|
|
#include "call/packet_receiver.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// Private API that is fixing surface between DirectTransport and underlying
|
|
// network conditions simulation implementation.
|
|
class SimulatedPacketReceiverInterface : public PacketReceiver {
|
|
public:
|
|
// Must not be called in parallel with DeliverPacket or Process.
|
|
// Destination receiver will be injected with this method
|
|
virtual void SetReceiver(PacketReceiver* receiver) = 0;
|
|
|
|
// Reports average packet delay.
|
|
virtual int AverageDelay() = 0;
|
|
|
|
// Process any pending tasks such as timeouts.
|
|
// Called on a worker thread.
|
|
virtual void Process() = 0;
|
|
|
|
// Returns the time until next process or nullopt to indicate that the next
|
|
// process time is unknown. If the next process time is unknown, this should
|
|
// be checked again any time a packet is enqueued.
|
|
virtual absl::optional<int64_t> TimeUntilNextProcess() = 0;
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // CALL_SIMULATED_PACKET_RECEIVER_H_
|