mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
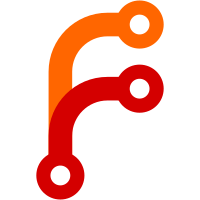
Current implementation has mouse cursor as part of the screen itself which means that everytime a cursor changes location, we have to update whole screen content, which brings unnecessary load overhead. Using our own mouse cursor monitor implementation allows us to track only mouse cursor changes and update them separately for much better performance. Bug: webrtc:13429 Change-Id: I224e9145f0bc7e45eafe4490de160f2ad4c8b545 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/244507 Reviewed-by: Tomas Gunnarsson <tommi@webrtc.org> Reviewed-by: Mark Foltz <mfoltz@chromium.org> Commit-Queue: Mark Foltz <mfoltz@chromium.org> Cr-Commit-Position: refs/heads/main@{#36011}
65 lines
1.9 KiB
C++
65 lines
1.9 KiB
C++
/*
|
|
* Copyright 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include <memory>
|
|
|
|
#include "modules/desktop_capture/desktop_capture_types.h"
|
|
#include "modules/desktop_capture/mouse_cursor_monitor.h"
|
|
|
|
#if defined(WEBRTC_USE_X11)
|
|
#include "modules/desktop_capture/linux/x11/mouse_cursor_monitor_x11.h"
|
|
#endif // defined(WEBRTC_USE_X11)
|
|
|
|
#if defined(WEBRTC_USE_PIPEWIRE)
|
|
#include "modules/desktop_capture/linux/wayland/mouse_cursor_monitor_pipewire.h"
|
|
#endif // defined(WEBRTC_USE_PIPEWIRE)
|
|
|
|
namespace webrtc {
|
|
|
|
// static
|
|
MouseCursorMonitor* MouseCursorMonitor::CreateForWindow(
|
|
const DesktopCaptureOptions& options,
|
|
WindowId window) {
|
|
#if defined(WEBRTC_USE_X11)
|
|
return MouseCursorMonitorX11::CreateForWindow(options, window);
|
|
#else
|
|
return nullptr;
|
|
#endif // defined(WEBRTC_USE_X11)
|
|
}
|
|
|
|
// static
|
|
MouseCursorMonitor* MouseCursorMonitor::CreateForScreen(
|
|
const DesktopCaptureOptions& options,
|
|
ScreenId screen) {
|
|
#if defined(WEBRTC_USE_X11)
|
|
return MouseCursorMonitorX11::CreateForScreen(options, screen);
|
|
#else
|
|
return nullptr;
|
|
#endif // defined(WEBRTC_USE_X11)
|
|
}
|
|
|
|
// static
|
|
std::unique_ptr<MouseCursorMonitor> MouseCursorMonitor::Create(
|
|
const DesktopCaptureOptions& options) {
|
|
#if defined(WEBRTC_USE_PIPEWIRE)
|
|
if (options.allow_pipewire() && DesktopCapturer::IsRunningUnderWayland() &&
|
|
options.screencast_stream()) {
|
|
return std::make_unique<MouseCursorMonitorPipeWire>(options);
|
|
}
|
|
#endif // defined(WEBRTC_USE_PIPEWIRE)
|
|
|
|
#if defined(WEBRTC_USE_X11)
|
|
return MouseCursorMonitorX11::Create(options);
|
|
#else
|
|
return nullptr;
|
|
#endif // defined(WEBRTC_USE_X11)
|
|
}
|
|
|
|
} // namespace webrtc
|