mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
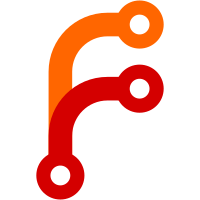
Packets, chunks, parameters and error causes - the SCTP entities that are sent on the wire - are buffers with fields that are stored in big endian and that generally consist of a fixed header size, and a variable sized part, that can e.g. be encoded sub-fields or serialized strings. The BoundedByteReader and BoundedByteWriter utilities make it easy to read those fields with as much aid from the compiler as possible, by having compile-time assertions that fields are not accessed outside the buffer's span. There are some byte reading functionality already in modules/rtp_rtcp, but that module would be a bit unfortunate to depend on, and doesn't have the compile time bounds checking that is the biggest feature of this abstraction of an rtc::ArrayView. Bug: webrtc:12614 Change-Id: I9fc641aff22221018dda9add4e2c44853c0f64f0 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/212967 Commit-Queue: Victor Boivie <boivie@webrtc.org> Reviewed-by: Mirko Bonadei <mbonadei@webrtc.org> Reviewed-by: Tommi <tommi@webrtc.org> Cr-Commit-Position: refs/heads/master@{#33597}
48 lines
1.3 KiB
C++
48 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2021 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "net/dcsctp/packet/bounded_byte_writer.h"
|
|
|
|
#include <vector>
|
|
|
|
#include "api/array_view.h"
|
|
#include "rtc_base/buffer.h"
|
|
#include "rtc_base/checks.h"
|
|
#include "rtc_base/gunit.h"
|
|
#include "test/gmock.h"
|
|
|
|
namespace dcsctp {
|
|
namespace {
|
|
using ::testing::ElementsAre;
|
|
|
|
TEST(BoundedByteWriterTest, CanWriteData) {
|
|
std::vector<uint8_t> data(14);
|
|
|
|
BoundedByteWriter<8> writer(data);
|
|
writer.Store32<0>(0x01020304);
|
|
writer.Store16<4>(0x0506);
|
|
writer.Store8<6>(0x07);
|
|
writer.Store8<7>(0x08);
|
|
|
|
uint8_t variable_data[] = {0, 0, 0, 0, 3, 0};
|
|
writer.CopyToVariableData(variable_data);
|
|
|
|
BoundedByteWriter<6> sub = writer.sub_writer<6>(0);
|
|
sub.Store32<0>(0x09000000);
|
|
sub.Store16<2>(0x0102);
|
|
|
|
BoundedByteWriter<2> sub2 = writer.sub_writer<2>(4);
|
|
sub2.Store8<1>(0x04);
|
|
|
|
EXPECT_THAT(data, ElementsAre(1, 2, 3, 4, 5, 6, 7, 8, 9, 0, 1, 2, 3, 4));
|
|
}
|
|
|
|
} // namespace
|
|
} // namespace dcsctp
|