mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
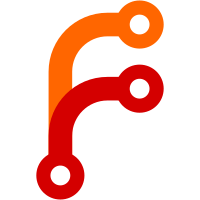
The timestamps returned by the clocks do not have an epoch. Each clock should be able to convert a timestamp it returns to an NTP time. The default implementation for querying for an NTP time is converting the current timestamp. This is favored over returning the offset between the relative and the NTP time because there is a field trial that makes the real clock revert to using system dependent methods for getting the NTP time. Bug: webrtc:11327 Change-Id: Ia139b2744b407cae94420bf9112212ec577efb16 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/219687 Reviewed-by: Minyue Li <minyue@webrtc.org> Reviewed-by: Niels Moller <nisse@webrtc.org> Commit-Queue: Paul Hallak <phallak@google.com> Cr-Commit-Position: refs/heads/master@{#34071}
45 lines
1.4 KiB
C++
45 lines
1.4 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "test/drifting_clock.h"
|
|
|
|
#include "rtc_base/checks.h"
|
|
|
|
namespace webrtc {
|
|
namespace test {
|
|
constexpr float DriftingClock::kNoDrift;
|
|
|
|
DriftingClock::DriftingClock(Clock* clock, float speed)
|
|
: clock_(clock), drift_(speed - 1.0f), start_time_(clock_->CurrentTime()) {
|
|
RTC_CHECK(clock);
|
|
RTC_CHECK_GT(speed, 0.0f);
|
|
}
|
|
|
|
TimeDelta DriftingClock::Drift() const {
|
|
auto now = clock_->CurrentTime();
|
|
RTC_DCHECK_GE(now, start_time_);
|
|
return (now - start_time_) * drift_;
|
|
}
|
|
|
|
Timestamp DriftingClock::Drift(Timestamp timestamp) const {
|
|
return timestamp + Drift() / 1000.;
|
|
}
|
|
|
|
NtpTime DriftingClock::Drift(NtpTime ntp_time) const {
|
|
// NTP precision is 1/2^32 seconds, i.e. 2^32 ntp fractions = 1 second.
|
|
const double kNtpFracPerMicroSecond = 4294.967296; // = 2^32 / 10^6
|
|
|
|
uint64_t total_fractions = static_cast<uint64_t>(ntp_time);
|
|
total_fractions += Drift().us() * kNtpFracPerMicroSecond;
|
|
return NtpTime(total_fractions);
|
|
}
|
|
|
|
} // namespace test
|
|
} // namespace webrtc
|