mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
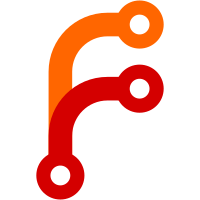
* FrameCombiner is simpler. No additional channel pointers for buffers. * Improve consistency in using views in downstream classes. * Deprecate older methods (some have upstream dependencies). * Use samples per channel instead of sample rate where the former is really what's needed. Bug: chromium:335805780 Change-Id: I0dde8ed7a5a187bbddd18d3b6c649aa0865e6d4a Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/352582 Commit-Queue: Tomas Gunnarsson <tommi@webrtc.org> Reviewed-by: Sam Zackrisson <saza@webrtc.org> Cr-Commit-Position: refs/heads/main@{#42575}
62 lines
2 KiB
C++
62 lines
2 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "modules/audio_processing/agc2/limiter.h"
|
|
|
|
#include <algorithm>
|
|
|
|
#include "common_audio/include/audio_util.h"
|
|
#include "modules/audio_processing/agc2/agc2_common.h"
|
|
#include "modules/audio_processing/agc2/agc2_testing_common.h"
|
|
#include "modules/audio_processing/agc2/vector_float_frame.h"
|
|
#include "modules/audio_processing/logging/apm_data_dumper.h"
|
|
#include "rtc_base/gunit.h"
|
|
|
|
namespace webrtc {
|
|
|
|
TEST(Limiter, LimiterShouldConstructAndRun) {
|
|
constexpr size_t kSamplesPerChannel = 480;
|
|
ApmDataDumper apm_data_dumper(0);
|
|
|
|
Limiter limiter(&apm_data_dumper, kSamplesPerChannel, "");
|
|
|
|
std::array<float, kSamplesPerChannel> buffer;
|
|
buffer.fill(kMaxAbsFloatS16Value);
|
|
limiter.Process(
|
|
DeinterleavedView<float>(buffer.data(), kSamplesPerChannel, 1));
|
|
}
|
|
|
|
TEST(Limiter, OutputVolumeAboveThreshold) {
|
|
constexpr size_t kSamplesPerChannel = 480;
|
|
const float input_level =
|
|
(kMaxAbsFloatS16Value + DbfsToFloatS16(test::kLimiterMaxInputLevelDbFs)) /
|
|
2.f;
|
|
ApmDataDumper apm_data_dumper(0);
|
|
|
|
Limiter limiter(&apm_data_dumper, kSamplesPerChannel, "");
|
|
|
|
std::array<float, kSamplesPerChannel> buffer;
|
|
|
|
// Give the level estimator time to adapt.
|
|
for (int i = 0; i < 5; ++i) {
|
|
std::fill(buffer.begin(), buffer.end(), input_level);
|
|
limiter.Process(
|
|
DeinterleavedView<float>(buffer.data(), kSamplesPerChannel, 1));
|
|
}
|
|
|
|
std::fill(buffer.begin(), buffer.end(), input_level);
|
|
limiter.Process(
|
|
DeinterleavedView<float>(buffer.data(), kSamplesPerChannel, 1));
|
|
for (const auto& sample : buffer) {
|
|
ASSERT_LT(0.9f * kMaxAbsFloatS16Value, sample);
|
|
}
|
|
}
|
|
|
|
} // namespace webrtc
|