mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
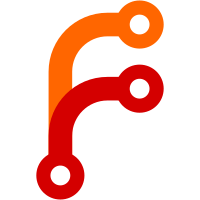
Original change's description:
> Revert "Use backticks not vertical bars to denote variables in comments for /pc"
>
> This reverts commit 37ee0f5e59
.
>
> Reason for revert: Revert in order to be able to revert https://webrtc-review.googlesource.com/c/src/+/225642
>
> Original change's description:
> > Use backticks not vertical bars to denote variables in comments for /pc
> >
> > Bug: webrtc:12338
> > Change-Id: I88cf10afa5fc810b95d2a585ab2e895dcc163b63
> > Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/226953
> > Reviewed-by: Harald Alvestrand <hta@webrtc.org>
> > Commit-Queue: Artem Titov <titovartem@webrtc.org>
> > Cr-Commit-Position: refs/heads/master@{#34575}
>
> TBR=hta@webrtc.org,titovartem@webrtc.org,webrtc-scoped@luci-project-accounts.iam.gserviceaccount.com
>
> Change-Id: I5eddd3a14e1f664bf831e5c294fbc4de5f6a88af
> No-Presubmit: true
> No-Tree-Checks: true
> No-Try: true
> Bug: webrtc:12338
> Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/227082
> Reviewed-by: Björn Terelius <terelius@webrtc.org>
> Commit-Queue: Björn Terelius <terelius@webrtc.org>
> Cr-Commit-Position: refs/heads/master@{#34577}
Bug: webrtc:12338
Change-Id: I96bd229b73613c162d11d75fa4f5934e1b4295c7
Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/227087
Commit-Queue: Artem Titov <titovartem@webrtc.org>
Reviewed-by: Harald Alvestrand <hta@webrtc.org>
Cr-Commit-Position: refs/heads/master@{#34611}
44 lines
1.7 KiB
C++
44 lines
1.7 KiB
C++
/*
|
|
* Copyright 2018 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef PC_RTC_STATS_TRAVERSAL_H_
|
|
#define PC_RTC_STATS_TRAVERSAL_H_
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "api/scoped_refptr.h"
|
|
#include "api/stats/rtc_stats.h"
|
|
#include "api/stats/rtc_stats_report.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// Traverses the stats graph, taking all stats objects that are directly or
|
|
// indirectly accessible from and including the stats objects identified by
|
|
// `ids`, returning them as a new stats report.
|
|
// This is meant to be used to implement the stats selection algorithm.
|
|
// https://w3c.github.io/webrtc-pc/#dfn-stats-selection-algorithm
|
|
rtc::scoped_refptr<RTCStatsReport> TakeReferencedStats(
|
|
rtc::scoped_refptr<RTCStatsReport> report,
|
|
const std::vector<std::string>& ids);
|
|
|
|
// Gets pointers to the string values of any members in `stats` that are used as
|
|
// references for looking up other stats objects in the same report by ID. The
|
|
// pointers are valid for the lifetime of `stats` assumings its members are not
|
|
// modified.
|
|
//
|
|
// For example, RTCCodecStats contains "transportId"
|
|
// (RTCCodecStats::transport_id) referencing an RTCTransportStats.
|
|
// https://w3c.github.io/webrtc-stats/#dom-rtccodecstats-transportid
|
|
std::vector<const std::string*> GetStatsReferencedIds(const RTCStats& stats);
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // PC_RTC_STATS_TRAVERSAL_H_
|