mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
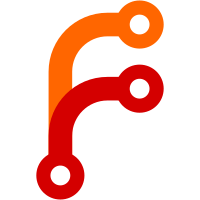
This is to ensure Epoch is the same if transport switch to TCP or another transport. First packet received will always be timestamped with rtc::TimeMicros. Other packet timstamps will use the kernel timestamp as an offset from the first packet timestamp. For BWE, it is important that there is not a large time base diff if transport change. This change is protected by the experiment WebRTC-SCM-Timestamp. Bug: webrtc:14066 Change-Id: Iaeb49831e7019e21601bc90895ac56003a54e206 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/281000 Reviewed-by: Evan Shrubsole <eshr@webrtc.org> Reviewed-by: Tomas Gunnarsson <tommi@webrtc.org> Commit-Queue: Per Kjellander <perkj@webrtc.org> Cr-Commit-Position: refs/heads/main@{#38587}
77 lines
2.7 KiB
C++
77 lines
2.7 KiB
C++
/*
|
|
* Copyright 2004 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef RTC_BASE_ASYNC_UDP_SOCKET_H_
|
|
#define RTC_BASE_ASYNC_UDP_SOCKET_H_
|
|
|
|
#include <stddef.h>
|
|
|
|
#include <cstdint>
|
|
#include <memory>
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "api/sequence_checker.h"
|
|
#include "rtc_base/async_packet_socket.h"
|
|
#include "rtc_base/socket.h"
|
|
#include "rtc_base/socket_address.h"
|
|
#include "rtc_base/socket_factory.h"
|
|
#include "rtc_base/thread_annotations.h"
|
|
|
|
namespace rtc {
|
|
|
|
// Provides the ability to receive packets asynchronously. Sends are not
|
|
// buffered since it is acceptable to drop packets under high load.
|
|
class AsyncUDPSocket : public AsyncPacketSocket {
|
|
public:
|
|
// Binds `socket` and creates AsyncUDPSocket for it. Takes ownership
|
|
// of `socket`. Returns null if bind() fails (`socket` is destroyed
|
|
// in that case).
|
|
static AsyncUDPSocket* Create(Socket* socket,
|
|
const SocketAddress& bind_address);
|
|
// Creates a new socket for sending asynchronous UDP packets using an
|
|
// asynchronous socket from the given factory.
|
|
static AsyncUDPSocket* Create(SocketFactory* factory,
|
|
const SocketAddress& bind_address);
|
|
explicit AsyncUDPSocket(Socket* socket);
|
|
~AsyncUDPSocket() = default;
|
|
|
|
SocketAddress GetLocalAddress() const override;
|
|
SocketAddress GetRemoteAddress() const override;
|
|
int Send(const void* pv,
|
|
size_t cb,
|
|
const rtc::PacketOptions& options) override;
|
|
int SendTo(const void* pv,
|
|
size_t cb,
|
|
const SocketAddress& addr,
|
|
const rtc::PacketOptions& options) override;
|
|
int Close() override;
|
|
|
|
State GetState() const override;
|
|
int GetOption(Socket::Option opt, int* value) override;
|
|
int SetOption(Socket::Option opt, int value) override;
|
|
int GetError() const override;
|
|
void SetError(int error) override;
|
|
|
|
private:
|
|
// Called when the underlying socket is ready to be read from.
|
|
void OnReadEvent(Socket* socket);
|
|
// Called when the underlying socket is ready to send.
|
|
void OnWriteEvent(Socket* socket);
|
|
|
|
RTC_NO_UNIQUE_ADDRESS webrtc::SequenceChecker sequence_checker_;
|
|
std::unique_ptr<Socket> socket_;
|
|
static constexpr int BUF_SIZE = 64 * 1024;
|
|
char buf_[BUF_SIZE] RTC_GUARDED_BY(sequence_checker_);
|
|
absl::optional<int64_t> socket_time_offset_ RTC_GUARDED_BY(sequence_checker_);
|
|
};
|
|
|
|
} // namespace rtc
|
|
|
|
#endif // RTC_BASE_ASYNC_UDP_SOCKET_H_
|