mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
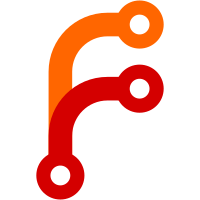
Currently test code passes pointer to temporary objects, while RtcpSender passes raw pointers to objects that are then seen as owned, and will be manually deleted by a overloaded destructor, which is scary and fragile. This CL moves all usage to std::unique_ptr<RtcpPacket> instead, which may create some heap churn in unit tests but that should be fine. Bug: webrtc:11925 Change-Id: I981bc7ccd6a74115c5a3de64b8427adbf3f16cc7 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/183920 Commit-Queue: Erik Språng <sprang@webrtc.org> Reviewed-by: Danil Chapovalov <danilchap@webrtc.org> Cr-Commit-Position: refs/heads/master@{#32084}
50 lines
1.4 KiB
C++
50 lines
1.4 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "modules/rtp_rtcp/source/rtcp_packet/compound_packet.h"
|
|
|
|
#include <memory>
|
|
#include <utility>
|
|
|
|
#include "rtc_base/checks.h"
|
|
|
|
namespace webrtc {
|
|
namespace rtcp {
|
|
|
|
CompoundPacket::CompoundPacket() = default;
|
|
|
|
CompoundPacket::~CompoundPacket() = default;
|
|
|
|
void CompoundPacket::Append(std::unique_ptr<RtcpPacket> packet) {
|
|
RTC_CHECK(packet);
|
|
appended_packets_.push_back(std::move(packet));
|
|
}
|
|
|
|
bool CompoundPacket::Create(uint8_t* packet,
|
|
size_t* index,
|
|
size_t max_length,
|
|
PacketReadyCallback callback) const {
|
|
for (const auto& appended : appended_packets_) {
|
|
if (!appended->Create(packet, index, max_length, callback))
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
size_t CompoundPacket::BlockLength() const {
|
|
size_t block_length = 0;
|
|
for (const auto& appended : appended_packets_) {
|
|
block_length += appended->BlockLength();
|
|
}
|
|
return block_length;
|
|
}
|
|
|
|
} // namespace rtcp
|
|
} // namespace webrtc
|