mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-20 00:57:49 +01:00
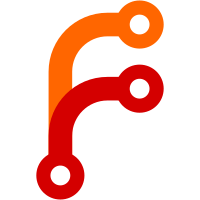
This change adds UMA stats that record the format of the remote offered SDP. There are three classifications for the SDP format: - Simple: No more than one audio and one video. Should be compatible with both Plan B and Unified Plan endpoints. - ComplexPlanB: More than one audio or more than one video in the Plan B format (e.g., one audio mline with multiple streams). - ComplexUnifiedPlan: More than one audio or more than one video in the Unified Plan format (e.g., two audio mlines). Bug: chromium:811683 Change-Id: If46394edfa6a812ef313d632e27ec27516c18867 Reviewed-on: https://webrtc-review.googlesource.com/57220 Commit-Queue: Steve Anton <steveanton@webrtc.org> Reviewed-by: Taylor Brandstetter <deadbeef@webrtc.org> Reviewed-by: Harald Alvestrand <hta@webrtc.org> Cr-Commit-Position: refs/heads/master@{#22315}
57 lines
1.9 KiB
C++
57 lines
1.9 KiB
C++
/*
|
|
* Copyright 2015 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef API_FAKEMETRICSOBSERVER_H_
|
|
#define API_FAKEMETRICSOBSERVER_H_
|
|
|
|
#include <map>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "api/peerconnectioninterface.h"
|
|
#include "rtc_base/thread_checker.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class FakeMetricsObserver : public MetricsObserverInterface {
|
|
public:
|
|
FakeMetricsObserver();
|
|
void Reset();
|
|
|
|
void IncrementEnumCounter(PeerConnectionEnumCounterType,
|
|
int counter,
|
|
int counter_max) override;
|
|
void AddHistogramSample(PeerConnectionMetricsName type,
|
|
int value) override;
|
|
|
|
// Accessors to be used by the tests.
|
|
int GetEnumCounter(PeerConnectionEnumCounterType type, int counter) const;
|
|
int GetHistogramSample(PeerConnectionMetricsName type) const;
|
|
|
|
// Returns true if and only if there is a count of 1 for the given counter and
|
|
// a count of 0 for all other counters of the given enum type.
|
|
bool ExpectOnlySingleEnumCount(PeerConnectionEnumCounterType type,
|
|
int counter) const;
|
|
|
|
protected:
|
|
~FakeMetricsObserver() {}
|
|
|
|
private:
|
|
rtc::ThreadChecker thread_checker_;
|
|
// The vector contains maps for each counter type. In the map, it's a mapping
|
|
// from individual counter to its count, such that it's memory efficient when
|
|
// comes to sparse enum types, like the SSL ciphers in the IANA registry.
|
|
std::vector<std::map<int, int>> counters_;
|
|
int histogram_samples_[kPeerConnectionMetricsName_Max];
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // API_FAKEMETRICSOBSERVER_H_
|