mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-15 06:40:43 +01:00
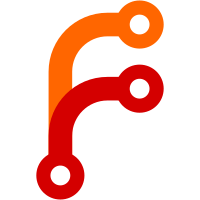
The VPN adapter type is not effectively supported in WebRTC Android for 1) the network monitor may not obtain the VPN adapter type from the OS, e.g. via NetworkInfo.getType, 2) and VPN adapter type is replaced by the adapter type of an underlying network by the network monitor in the current implementation. Specifically, WebRTC Android would previously classify VPNs as either type ADAPTER_TYPE_UNKNOWN, or the type of the currently active network (which we assume the VPN is using). In this CL, VPNs are classified as ADAPTER_TYPE_VPN whenever possible, and the underlying network type, if available from the VPN, is separately stored and used to prioritize ICE candidates in network path selection. This allows ADAPTER_TYPE_VPN to be used in networkIgnoreMask to ignore VPNs when gathering ICE candidates. Bug: webrtc:9168 Change-Id: I9513c76a114ba967437b699e71223a4a2f13f34a Reviewed-on: https://webrtc-review.googlesource.com/70960 Commit-Queue: Qingsi Wang <qingsi@google.com> Reviewed-by: Taylor Brandstetter <deadbeef@webrtc.org> Reviewed-by: Sami Kalliomäki <sakal@webrtc.org> Cr-Commit-Position: refs/heads/master@{#23061}
67 lines
2 KiB
C++
67 lines
2 KiB
C++
/*
|
|
* Copyright 2015 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "rtc_base/networkmonitor.h"
|
|
|
|
#include "rtc_base/checks.h"
|
|
|
|
namespace {
|
|
const uint32_t UPDATE_NETWORKS_MESSAGE = 1;
|
|
|
|
// This is set by NetworkMonitorFactory::SetFactory and the caller of
|
|
// NetworkMonitorFactory::SetFactory must be responsible for calling
|
|
// ReleaseFactory to destroy the factory.
|
|
rtc::NetworkMonitorFactory* network_monitor_factory = nullptr;
|
|
} // namespace
|
|
|
|
namespace rtc {
|
|
NetworkMonitorInterface::NetworkMonitorInterface() {}
|
|
|
|
NetworkMonitorInterface::~NetworkMonitorInterface() {}
|
|
|
|
NetworkMonitorBase::NetworkMonitorBase() : worker_thread_(Thread::Current()) {}
|
|
NetworkMonitorBase::~NetworkMonitorBase() {}
|
|
|
|
void NetworkMonitorBase::OnNetworksChanged() {
|
|
RTC_LOG(LS_VERBOSE) << "Network change is received at the network monitor";
|
|
worker_thread_->Post(RTC_FROM_HERE, this, UPDATE_NETWORKS_MESSAGE);
|
|
}
|
|
|
|
void NetworkMonitorBase::OnMessage(Message* msg) {
|
|
RTC_DCHECK(msg->message_id == UPDATE_NETWORKS_MESSAGE);
|
|
SignalNetworksChanged();
|
|
}
|
|
|
|
AdapterType NetworkMonitorBase::GetVpnUnderlyingAdapterType(
|
|
const std::string& interface_name) {
|
|
return ADAPTER_TYPE_UNKNOWN;
|
|
}
|
|
|
|
NetworkMonitorFactory::NetworkMonitorFactory() {}
|
|
NetworkMonitorFactory::~NetworkMonitorFactory() {}
|
|
|
|
void NetworkMonitorFactory::SetFactory(NetworkMonitorFactory* factory) {
|
|
if (network_monitor_factory != nullptr) {
|
|
delete network_monitor_factory;
|
|
}
|
|
network_monitor_factory = factory;
|
|
}
|
|
|
|
void NetworkMonitorFactory::ReleaseFactory(NetworkMonitorFactory* factory) {
|
|
if (factory == network_monitor_factory) {
|
|
SetFactory(nullptr);
|
|
}
|
|
}
|
|
|
|
NetworkMonitorFactory* NetworkMonitorFactory::GetFactory() {
|
|
return network_monitor_factory;
|
|
}
|
|
|
|
} // namespace rtc
|