mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-15 06:40:43 +01:00
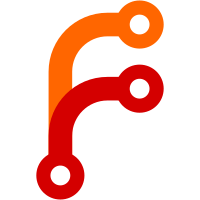
This reverts commit43d069a2cd
. Reason for revert: Include fix for local reference overflow. Original change's description: > Revert "Android: Generate JNI code for stats" > > This reverts commitaede67a199
. > > Reason for revert: Causes error: > JNI ERROR (app bug): local reference table overflow (max=512)' > > Original change's description: > > Android: Generate JNI code for stats > > > > This CL also unifies the functions for converting from C++ to Java, and > > generates the boiler plate for converting C++ vectors to Java arrays. > > > > Bug: webrtc:8278 > > Change-Id: I262e9162beae8a64ba0e8b6a27e1081207b03961 > > Reviewed-on: https://webrtc-review.googlesource.com/26020 > > Commit-Queue: Magnus Jedvert <magjed@webrtc.org> > > Reviewed-by: Sami Kalliomäki <sakal@webrtc.org> > > Cr-Commit-Position: refs/heads/master@{#20918} > > TBR=magjed@webrtc.org,sakal@webrtc.org > > Change-Id: Ieb26ed8577bd489a4dd4f7542d16a7d0e11f409f > No-Presubmit: true > No-Tree-Checks: true > No-Try: true > Bug: webrtc:8278 > Reviewed-on: https://webrtc-review.googlesource.com/26900 > Reviewed-by: Magnus Jedvert <magjed@webrtc.org> > Commit-Queue: Magnus Jedvert <magjed@webrtc.org> > Cr-Commit-Position: refs/heads/master@{#20926} TBR=magjed@webrtc.org,sakal@webrtc.org Change-Id: I6f7097f308098e7922fbf0bed577bd69da4e1c61 Bug: webrtc:8278 Reviewed-on: https://webrtc-review.googlesource.com/26901 Commit-Queue: Magnus Jedvert <magjed@webrtc.org> Reviewed-by: Magnus Jedvert <magjed@webrtc.org> Cr-Commit-Position: refs/heads/master@{#20951}
52 lines
1.3 KiB
Java
52 lines
1.3 KiB
Java
/*
|
|
* Copyright 2013 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
package org.webrtc;
|
|
|
|
/**
|
|
* Representation of a single ICE Candidate, mirroring
|
|
* {@code IceCandidateInterface} in the C++ API.
|
|
*/
|
|
public class IceCandidate {
|
|
public final String sdpMid;
|
|
public final int sdpMLineIndex;
|
|
public final String sdp;
|
|
public final String serverUrl;
|
|
|
|
public IceCandidate(String sdpMid, int sdpMLineIndex, String sdp) {
|
|
this.sdpMid = sdpMid;
|
|
this.sdpMLineIndex = sdpMLineIndex;
|
|
this.sdp = sdp;
|
|
this.serverUrl = "";
|
|
}
|
|
|
|
@CalledByNative
|
|
IceCandidate(String sdpMid, int sdpMLineIndex, String sdp, String serverUrl) {
|
|
this.sdpMid = sdpMid;
|
|
this.sdpMLineIndex = sdpMLineIndex;
|
|
this.sdp = sdp;
|
|
this.serverUrl = serverUrl;
|
|
}
|
|
|
|
@Override
|
|
public String toString() {
|
|
return sdpMid + ":" + sdpMLineIndex + ":" + sdp + ":" + serverUrl;
|
|
}
|
|
|
|
@CalledByNative
|
|
String getSdpMid() {
|
|
return sdpMid;
|
|
}
|
|
|
|
@CalledByNative
|
|
String getSdp() {
|
|
return sdp;
|
|
}
|
|
}
|