mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-17 07:37:51 +01:00
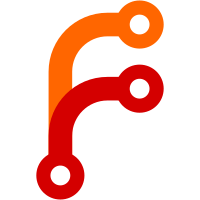
Adds the types and methods required for sending and receiving data channel messages over the media transport. These are: - A DataMessageType to distinguish between text, binary, and control messages - A parameters struct for sending data messages, which specifies the channel id, type, and ordering/reliability parameters - A sink for data-channel related callbacks (receive data, begin closing procedure, and end closing procedure) - A method to set the sink for data channels - Methods to open, close, and send on data channels These methods, combined with the state sink, allow PeerConnection to implement the DataChannelProviderInterface using MediaTransport as the underlying transport. Change-Id: Iccb2ba374594762a5b4f995564e2a1ff7d8805f5 Bug: webrtc:9719 Reviewed-on: https://webrtc-review.googlesource.com/c/108541 Reviewed-by: Anton Sukhanov <sukhanov@webrtc.org> Reviewed-by: Niels Moller <nisse@webrtc.org> Reviewed-by: Seth Hampson <shampson@webrtc.org> Reviewed-by: Peter Slatala <psla@webrtc.org> Commit-Queue: Bjorn Mellem <mellem@webrtc.org> Cr-Commit-Position: refs/heads/master@{#25454}
119 lines
4.2 KiB
C++
119 lines
4.2 KiB
C++
/*
|
|
* Copyright 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
// This is EXPERIMENTAL interface for media transport.
|
|
//
|
|
// The goal is to refactor WebRTC code so that audio and video frames
|
|
// are sent / received through the media transport interface. This will
|
|
// enable different media transport implementations, including QUIC-based
|
|
// media transport.
|
|
|
|
#include "api/media_transport_interface.h"
|
|
|
|
#include <cstdint>
|
|
#include <utility>
|
|
|
|
namespace webrtc {
|
|
|
|
MediaTransportSettings::MediaTransportSettings() = default;
|
|
MediaTransportSettings::MediaTransportSettings(const MediaTransportSettings&) =
|
|
default;
|
|
MediaTransportSettings& MediaTransportSettings::operator=(
|
|
const MediaTransportSettings&) = default;
|
|
MediaTransportSettings::~MediaTransportSettings() = default;
|
|
|
|
MediaTransportEncodedAudioFrame::~MediaTransportEncodedAudioFrame() {}
|
|
|
|
MediaTransportEncodedAudioFrame::MediaTransportEncodedAudioFrame(
|
|
int sampling_rate_hz,
|
|
int starting_sample_index,
|
|
int samples_per_channel,
|
|
int sequence_number,
|
|
FrameType frame_type,
|
|
uint8_t payload_type,
|
|
std::vector<uint8_t> encoded_data)
|
|
: sampling_rate_hz_(sampling_rate_hz),
|
|
starting_sample_index_(starting_sample_index),
|
|
samples_per_channel_(samples_per_channel),
|
|
sequence_number_(sequence_number),
|
|
frame_type_(frame_type),
|
|
payload_type_(payload_type),
|
|
encoded_data_(std::move(encoded_data)) {}
|
|
|
|
MediaTransportEncodedAudioFrame& MediaTransportEncodedAudioFrame::operator=(
|
|
const MediaTransportEncodedAudioFrame&) = default;
|
|
|
|
MediaTransportEncodedAudioFrame& MediaTransportEncodedAudioFrame::operator=(
|
|
MediaTransportEncodedAudioFrame&&) = default;
|
|
|
|
MediaTransportEncodedAudioFrame::MediaTransportEncodedAudioFrame(
|
|
const MediaTransportEncodedAudioFrame&) = default;
|
|
|
|
MediaTransportEncodedAudioFrame::MediaTransportEncodedAudioFrame(
|
|
MediaTransportEncodedAudioFrame&&) = default;
|
|
|
|
MediaTransportEncodedVideoFrame::~MediaTransportEncodedVideoFrame() {}
|
|
|
|
MediaTransportEncodedVideoFrame::MediaTransportEncodedVideoFrame(
|
|
int64_t frame_id,
|
|
std::vector<int64_t> referenced_frame_ids,
|
|
VideoCodecType codec_type,
|
|
const webrtc::EncodedImage& encoded_image)
|
|
: codec_type_(codec_type),
|
|
encoded_image_(encoded_image),
|
|
frame_id_(frame_id),
|
|
referenced_frame_ids_(std::move(referenced_frame_ids)) {}
|
|
|
|
MediaTransportEncodedVideoFrame& MediaTransportEncodedVideoFrame::operator=(
|
|
const MediaTransportEncodedVideoFrame&) = default;
|
|
|
|
MediaTransportEncodedVideoFrame& MediaTransportEncodedVideoFrame::operator=(
|
|
MediaTransportEncodedVideoFrame&&) = default;
|
|
|
|
MediaTransportEncodedVideoFrame::MediaTransportEncodedVideoFrame(
|
|
const MediaTransportEncodedVideoFrame&) = default;
|
|
|
|
MediaTransportEncodedVideoFrame::MediaTransportEncodedVideoFrame(
|
|
MediaTransportEncodedVideoFrame&&) = default;
|
|
|
|
SendDataParams::SendDataParams() = default;
|
|
|
|
RTCError MediaTransportInterface::SendData(
|
|
int channel_id,
|
|
const SendDataParams& params,
|
|
const rtc::CopyOnWriteBuffer& buffer) {
|
|
RTC_NOTREACHED();
|
|
return RTCError(RTCErrorType::UNSUPPORTED_OPERATION, "Not implemented");
|
|
}
|
|
|
|
RTCError MediaTransportInterface::CloseChannel(int channel_id) {
|
|
RTC_NOTREACHED();
|
|
return RTCError(RTCErrorType::UNSUPPORTED_OPERATION, "Not implemented");
|
|
}
|
|
|
|
RTCErrorOr<std::unique_ptr<MediaTransportInterface>>
|
|
MediaTransportFactory::CreateMediaTransport(
|
|
rtc::PacketTransportInternal* packet_transport,
|
|
rtc::Thread* network_thread,
|
|
bool is_caller) {
|
|
MediaTransportSettings settings;
|
|
settings.is_caller = is_caller;
|
|
return CreateMediaTransport(packet_transport, network_thread, settings);
|
|
}
|
|
|
|
RTCErrorOr<std::unique_ptr<MediaTransportInterface>>
|
|
MediaTransportFactory::CreateMediaTransport(
|
|
rtc::PacketTransportInternal* packet_transport,
|
|
rtc::Thread* network_thread,
|
|
const MediaTransportSettings& settings) {
|
|
return std::unique_ptr<MediaTransportInterface>(nullptr);
|
|
}
|
|
|
|
} // namespace webrtc
|