mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 14:20:45 +01:00
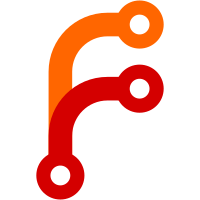
This is a reland of fa79081dca
It crashed due to inability to handle small timestamps in probe
estimator. This was fixed by moving history window check to avoid
subtracting from the timestamp.
Original change's description:
> Cleanup of RTP references in GoogCC implementation.
>
> As the send time congestion controller now has been removed,
> we don't need the RTP related constructs anymore.
>
> Bug: webrtc:9510
> Change-Id: I02c059ed8ae907ab4672d183c5639ad459b581aa
> Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/142221
> Commit-Queue: Sebastian Jansson <srte@webrtc.org>
> Reviewed-by: Björn Terelius <terelius@webrtc.org>
> Cr-Commit-Position: refs/heads/master@{#28330}
Bug: webrtc:9510
Change-Id: I3bf91222068e4fbb6aa159bfeb7a73e00bb6a0d7
Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/143165
Reviewed-by: Björn Terelius <terelius@webrtc.org>
Commit-Queue: Sebastian Jansson <srte@webrtc.org>
Cr-Commit-Position: refs/heads/master@{#28347}
61 lines
2 KiB
C++
61 lines
2 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_CONGESTION_CONTROLLER_GOOG_CC_PROBE_BITRATE_ESTIMATOR_H_
|
|
#define MODULES_CONGESTION_CONTROLLER_GOOG_CC_PROBE_BITRATE_ESTIMATOR_H_
|
|
|
|
#include <limits>
|
|
#include <map>
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "api/transport/network_types.h"
|
|
#include "api/units/data_rate.h"
|
|
|
|
namespace webrtc {
|
|
class RtcEventLog;
|
|
|
|
class ProbeBitrateEstimator {
|
|
public:
|
|
explicit ProbeBitrateEstimator(RtcEventLog* event_log);
|
|
~ProbeBitrateEstimator();
|
|
|
|
// Should be called for every probe packet we receive feedback about.
|
|
// Returns the estimated bitrate if the probe completes a valid cluster.
|
|
absl::optional<DataRate> HandleProbeAndEstimateBitrate(
|
|
const PacketResult& packet_feedback);
|
|
|
|
absl::optional<DataRate> FetchAndResetLastEstimatedBitrate();
|
|
|
|
absl::optional<DataRate> last_estimate() const;
|
|
|
|
private:
|
|
struct AggregatedCluster {
|
|
int num_probes = 0;
|
|
Timestamp first_send = Timestamp::PlusInfinity();
|
|
Timestamp last_send = Timestamp::MinusInfinity();
|
|
Timestamp first_receive = Timestamp::PlusInfinity();
|
|
Timestamp last_receive = Timestamp::MinusInfinity();
|
|
DataSize size_last_send = DataSize::Zero();
|
|
DataSize size_first_receive = DataSize::Zero();
|
|
DataSize size_total = DataSize::Zero();
|
|
};
|
|
|
|
// Erases old cluster data that was seen before |timestamp|.
|
|
void EraseOldClusters(Timestamp timestamp);
|
|
|
|
std::map<int, AggregatedCluster> clusters_;
|
|
RtcEventLog* const event_log_;
|
|
absl::optional<DataRate> estimated_data_rate_;
|
|
absl::optional<DataRate> last_estimate_;
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_CONGESTION_CONTROLLER_GOOG_CC_PROBE_BITRATE_ESTIMATOR_H_
|