mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-17 07:37:51 +01:00
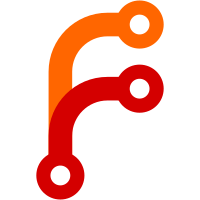
As the rate allocation has been moved into entirely into SimulcastRateAllocator, and the listeners are thus no longer needed, this class doesn't fill any other purpose than to determine if ScreenshareLayers or TemporalLayers should be created for a given simulcast stream. This can however be done just from looking at the VideoCodec instance, so changing this into a static factory method. Due to dependencies from upstream projects, keep the class name and field in VideoCodec around for now. Bug: webrtc:9012 Change-Id: I028fe6b2a19e0d16b35956cc2df01dcf5bfa7979 Reviewed-on: https://webrtc-review.googlesource.com/63264 Commit-Queue: Erik Språng <sprang@webrtc.org> Reviewed-by: Ilya Nikolaevskiy <ilnik@webrtc.org> Reviewed-by: Stefan Holmer <stefan@webrtc.org> Cr-Commit-Position: refs/heads/master@{#22529}
61 lines
2.1 KiB
C++
61 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_VIDEO_CODING_CODECS_VP8_SIMULCAST_RATE_ALLOCATOR_H_
|
|
#define MODULES_VIDEO_CODING_CODECS_VP8_SIMULCAST_RATE_ALLOCATOR_H_
|
|
|
|
#include <stdint.h>
|
|
|
|
#include <map>
|
|
#include <memory>
|
|
#include <vector>
|
|
|
|
#include "api/video_codecs/video_encoder.h"
|
|
#include "common_video/include/video_bitrate_allocator.h"
|
|
#include "modules/video_coding/codecs/vp8/temporal_layers.h"
|
|
#include "rtc_base/constructormagic.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class SimulcastRateAllocator : public VideoBitrateAllocator {
|
|
public:
|
|
explicit SimulcastRateAllocator(const VideoCodec& codec);
|
|
|
|
BitrateAllocation GetAllocation(uint32_t total_bitrate_bps,
|
|
uint32_t framerate) override;
|
|
uint32_t GetPreferredBitrateBps(uint32_t framerate) override;
|
|
const VideoCodec& GetCodec() const;
|
|
|
|
private:
|
|
void DistributeAllocationToSimulcastLayers(
|
|
uint32_t total_bitrate_bps,
|
|
BitrateAllocation* allocated_bitrates_bps) const;
|
|
void DistributeAllocationToTemporalLayers(
|
|
uint32_t framerate,
|
|
BitrateAllocation* allocated_bitrates_bps) const;
|
|
std::vector<uint32_t> DefaultTemporalLayerAllocation(int bitrate_kbps,
|
|
int max_bitrate_kbps,
|
|
int framerate,
|
|
int simulcast_id) const;
|
|
std::vector<uint32_t> ScreenshareTemporalLayerAllocation(
|
|
int bitrate_kbps,
|
|
int max_bitrate_kbps,
|
|
int framerate,
|
|
int simulcast_id) const;
|
|
int NumTemporalStreams(size_t simulcast_id) const;
|
|
|
|
const VideoCodec codec_;
|
|
|
|
RTC_DISALLOW_COPY_AND_ASSIGN(SimulcastRateAllocator);
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_VIDEO_CODING_CODECS_VP8_SIMULCAST_RATE_ALLOCATOR_H_
|