mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
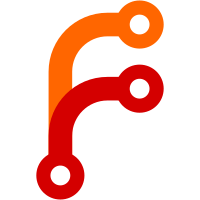
This provides the empty shell of an AudioGenerator class. It is intended to be used for debugging purposes and can be inserted into the APM much like an AecDump. It allows for playing out diagnostic audio unaffected by codecs and network jitter, while still capturing API interaction like in a normal call. NOTRY=True Bug: webrtc:8882 Change-Id: I8132afc95cdba02ab233f44e22e0a5f530710ef7 Reviewed-on: https://webrtc-review.googlesource.com/53300 Commit-Queue: Sam Zackrisson <saza@webrtc.org> Reviewed-by: Alex Loiko <aleloi@webrtc.org> Cr-Commit-Position: refs/heads/master@{#22282}
36 lines
1.3 KiB
C++
36 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_AUDIO_PROCESSING_INCLUDE_AUDIO_GENERATOR_H_
|
|
#define MODULES_AUDIO_PROCESSING_INCLUDE_AUDIO_GENERATOR_H_
|
|
|
|
#include "modules/audio_processing/include/audio_frame_view.h"
|
|
|
|
namespace webrtc {
|
|
// This class is used as input sink for the APM, for diagnostic purposes.
|
|
// Generates an infinite audio signal, [-1, 1] floating point values, in frames
|
|
// of fixed channel count and sample rate.
|
|
class AudioGenerator {
|
|
public:
|
|
virtual ~AudioGenerator() {}
|
|
|
|
// Fill |audio| with the next samples of the audio signal.
|
|
virtual void FillFrame(AudioFrameView<float> audio) = 0;
|
|
|
|
// Return the number of channels output by the AudioGenerator.
|
|
virtual size_t NumChannels() = 0;
|
|
|
|
// Return the sample rate output by the AudioGenerator.
|
|
virtual size_t SampleRateHz() = 0;
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_AUDIO_PROCESSING_INCLUDE_AUDIO_GENERATOR_H_
|