mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
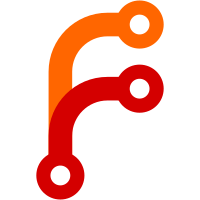
This change integrates fuzzing support for RtpDumps in WebRTC. This allows LibFuzzer to directly fuzz the RTP code path from packet arrival all the way to actual decoding and rendering. It does this by replaying each RTP packet in the RTPDump which can be mutated directly by the fuzzer. For fuzzing support the RtpFileReader needs to support reading from a buffer instead of an file. The test class requires FILE* for all its parsing operations and is deeply coupled this way. I chose to solve this problem at an OS level by using the tmpfile() option and copying the buffer to the tmpfile(). fmemopen() is no available on most platforms so couldn't be used as a generic solution. The additional copy isn't ideal but won't be a bottleneck for the fuzzing. In the future I plan for the fuzzers to read from a configuration file. But given the current packaging strategy for fuzzers in WebRTC this isn't easy. Bug: webrtc:9860 Change-Id: I2560120e82663f9e9fb5b9640e6a6d16f9c1a360 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/126682 Reviewed-by: Niels Moller <nisse@webrtc.org> Commit-Queue: Benjamin Wright <benwright@webrtc.org> Cr-Commit-Position: refs/heads/master@{#27151}
49 lines
1.6 KiB
C++
49 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2014 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#ifndef TEST_RTP_FILE_READER_H_
|
|
#define TEST_RTP_FILE_READER_H_
|
|
|
|
#include <set>
|
|
#include <string>
|
|
|
|
namespace webrtc {
|
|
namespace test {
|
|
|
|
struct RtpPacket {
|
|
// Accommodate for 50 ms packets of 32 kHz PCM16 samples (3200 bytes) plus
|
|
// some overhead.
|
|
static const size_t kMaxPacketBufferSize = 3500;
|
|
uint8_t data[kMaxPacketBufferSize];
|
|
size_t length;
|
|
// The length the packet had on wire. Will be different from |length| when
|
|
// reading a header-only RTP dump.
|
|
size_t original_length;
|
|
|
|
uint32_t time_ms;
|
|
};
|
|
|
|
class RtpFileReader {
|
|
public:
|
|
enum FileFormat { kPcap, kRtpDump, kLengthPacketInterleaved };
|
|
|
|
virtual ~RtpFileReader() {}
|
|
static RtpFileReader* Create(FileFormat format,
|
|
const uint8_t* data,
|
|
size_t size,
|
|
const std::set<uint32_t>& ssrc_filter);
|
|
static RtpFileReader* Create(FileFormat format, const std::string& filename);
|
|
static RtpFileReader* Create(FileFormat format,
|
|
const std::string& filename,
|
|
const std::set<uint32_t>& ssrc_filter);
|
|
virtual bool NextPacket(RtpPacket* packet) = 0;
|
|
};
|
|
} // namespace test
|
|
} // namespace webrtc
|
|
#endif // TEST_RTP_FILE_READER_H_
|