mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
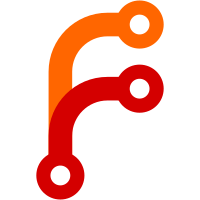
This reverts commit793bac569f
. Reason for revert: rare compilation error fixed Original change's description: > Revert "Refactor the PlatformThread API." > > This reverts commitc89fdd716c
. > > Reason for revert: Causes rare compilation error on win-libfuzzer-asan trybot. > See https://ci.chromium.org/p/chromium/builders/try/win-libfuzzer-asan-rel/713745? > > Original change's description: > > Refactor the PlatformThread API. > > > > PlatformThread's API is using old style function pointers, causes > > casting, is unintuitive and forces artificial call sequences, and > > is additionally possible to misuse in release mode. > > > > Fix this by an API face lift: > > 1. The class is turned into a handle, which can be empty. > > 2. The only way of getting a non-empty PlatformThread is by calling > > SpawnJoinable or SpawnDetached, clearly conveying the semantics to the > > code reader. > > 3. Handles can be Finalized, which works differently for joinable and > > detached threads: > > a) Handles for detached threads are simply closed where applicable. > > b) Joinable threads are joined before handles are closed. > > 4. The destructor finalizes handles. No explicit call is needed. > > > > Fixed: webrtc:12727 > > Change-Id: Id00a0464edf4fc9e552b6a1fbb5d2e1280e88811 > > Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/215075 > > Commit-Queue: Markus Handell <handellm@webrtc.org> > > Reviewed-by: Harald Alvestrand <hta@webrtc.org> > > Reviewed-by: Mirko Bonadei <mbonadei@webrtc.org> > > Reviewed-by: Tommi <tommi@webrtc.org> > > Cr-Commit-Position: refs/heads/master@{#33923} > > # Not skipping CQ checks because original CL landed > 1 day ago. > > TBR=handellm@webrtc.org > > Bug: webrtc:12727 > Change-Id: Ic0146be8866f6dd3ad9c364fb8646650b8e07419 > Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/217583 > Reviewed-by: Guido Urdaneta <guidou@webrtc.org> > Reviewed-by: Markus Handell <handellm@webrtc.org> > Commit-Queue: Guido Urdaneta <guidou@webrtc.org> > Cr-Commit-Position: refs/heads/master@{#33936} # Not skipping CQ checks because this is a reland. Bug: webrtc:12727 Change-Id: Ifd6f44eac72fed84474277a1be03eb84d2f4376e Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/217881 Commit-Queue: Mirko Bonadei <mbonadei@webrtc.org> Reviewed-by: Mirko Bonadei <mbonadei@webrtc.org> Reviewed-by: Markus Handell <handellm@webrtc.org> Reviewed-by: Harald Alvestrand <hta@webrtc.org> Cr-Commit-Position: refs/heads/master@{#33950}
208 lines
7.2 KiB
C++
208 lines
7.2 KiB
C++
/*
|
|
* Copyright (c) 2012 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef AUDIO_DEVICE_AUDIO_DEVICE_ALSA_LINUX_H_
|
|
#define AUDIO_DEVICE_AUDIO_DEVICE_ALSA_LINUX_H_
|
|
|
|
#include <memory>
|
|
|
|
#include "modules/audio_device/audio_device_generic.h"
|
|
#include "modules/audio_device/linux/audio_mixer_manager_alsa_linux.h"
|
|
#include "rtc_base/platform_thread.h"
|
|
#include "rtc_base/synchronization/mutex.h"
|
|
|
|
#if defined(WEBRTC_USE_X11)
|
|
#include <X11/Xlib.h>
|
|
#endif
|
|
#include <alsa/asoundlib.h>
|
|
#include <sys/ioctl.h>
|
|
#include <sys/soundcard.h>
|
|
|
|
typedef webrtc::adm_linux_alsa::AlsaSymbolTable WebRTCAlsaSymbolTable;
|
|
WebRTCAlsaSymbolTable* GetAlsaSymbolTable();
|
|
|
|
namespace webrtc {
|
|
|
|
class AudioDeviceLinuxALSA : public AudioDeviceGeneric {
|
|
public:
|
|
AudioDeviceLinuxALSA();
|
|
virtual ~AudioDeviceLinuxALSA();
|
|
|
|
// Retrieve the currently utilized audio layer
|
|
int32_t ActiveAudioLayer(
|
|
AudioDeviceModule::AudioLayer& audioLayer) const override;
|
|
|
|
// Main initializaton and termination
|
|
InitStatus Init() RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
int32_t Terminate() RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
bool Initialized() const override;
|
|
|
|
// Device enumeration
|
|
int16_t PlayoutDevices() override;
|
|
int16_t RecordingDevices() override;
|
|
int32_t PlayoutDeviceName(uint16_t index,
|
|
char name[kAdmMaxDeviceNameSize],
|
|
char guid[kAdmMaxGuidSize]) override;
|
|
int32_t RecordingDeviceName(uint16_t index,
|
|
char name[kAdmMaxDeviceNameSize],
|
|
char guid[kAdmMaxGuidSize]) override;
|
|
|
|
// Device selection
|
|
int32_t SetPlayoutDevice(uint16_t index) override;
|
|
int32_t SetPlayoutDevice(
|
|
AudioDeviceModule::WindowsDeviceType device) override;
|
|
int32_t SetRecordingDevice(uint16_t index) override;
|
|
int32_t SetRecordingDevice(
|
|
AudioDeviceModule::WindowsDeviceType device) override;
|
|
|
|
// Audio transport initialization
|
|
int32_t PlayoutIsAvailable(bool& available) override;
|
|
int32_t InitPlayout() RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
bool PlayoutIsInitialized() const override;
|
|
int32_t RecordingIsAvailable(bool& available) override;
|
|
int32_t InitRecording() RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
bool RecordingIsInitialized() const override;
|
|
|
|
// Audio transport control
|
|
int32_t StartPlayout() override;
|
|
int32_t StopPlayout() RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
bool Playing() const override;
|
|
int32_t StartRecording() override;
|
|
int32_t StopRecording() RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
bool Recording() const override;
|
|
|
|
// Audio mixer initialization
|
|
int32_t InitSpeaker() RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
bool SpeakerIsInitialized() const override;
|
|
int32_t InitMicrophone() RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
bool MicrophoneIsInitialized() const override;
|
|
|
|
// Speaker volume controls
|
|
int32_t SpeakerVolumeIsAvailable(bool& available) override;
|
|
int32_t SetSpeakerVolume(uint32_t volume) override;
|
|
int32_t SpeakerVolume(uint32_t& volume) const override;
|
|
int32_t MaxSpeakerVolume(uint32_t& maxVolume) const override;
|
|
int32_t MinSpeakerVolume(uint32_t& minVolume) const override;
|
|
|
|
// Microphone volume controls
|
|
int32_t MicrophoneVolumeIsAvailable(bool& available) override;
|
|
int32_t SetMicrophoneVolume(uint32_t volume) override;
|
|
int32_t MicrophoneVolume(uint32_t& volume) const override;
|
|
int32_t MaxMicrophoneVolume(uint32_t& maxVolume) const override;
|
|
int32_t MinMicrophoneVolume(uint32_t& minVolume) const override;
|
|
|
|
// Speaker mute control
|
|
int32_t SpeakerMuteIsAvailable(bool& available) override;
|
|
int32_t SetSpeakerMute(bool enable) override;
|
|
int32_t SpeakerMute(bool& enabled) const override;
|
|
|
|
// Microphone mute control
|
|
int32_t MicrophoneMuteIsAvailable(bool& available) override;
|
|
int32_t SetMicrophoneMute(bool enable) override;
|
|
int32_t MicrophoneMute(bool& enabled) const override;
|
|
|
|
// Stereo support
|
|
int32_t StereoPlayoutIsAvailable(bool& available)
|
|
RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
int32_t SetStereoPlayout(bool enable) override;
|
|
int32_t StereoPlayout(bool& enabled) const override;
|
|
int32_t StereoRecordingIsAvailable(bool& available)
|
|
RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
int32_t SetStereoRecording(bool enable) override;
|
|
int32_t StereoRecording(bool& enabled) const override;
|
|
|
|
// Delay information and control
|
|
int32_t PlayoutDelay(uint16_t& delayMS) const override;
|
|
|
|
void AttachAudioBuffer(AudioDeviceBuffer* audioBuffer)
|
|
RTC_LOCKS_EXCLUDED(mutex_) override;
|
|
|
|
private:
|
|
int32_t InitRecordingLocked() RTC_EXCLUSIVE_LOCKS_REQUIRED(mutex_);
|
|
int32_t StopRecordingLocked() RTC_EXCLUSIVE_LOCKS_REQUIRED(mutex_);
|
|
int32_t StopPlayoutLocked() RTC_EXCLUSIVE_LOCKS_REQUIRED(mutex_);
|
|
int32_t InitPlayoutLocked() RTC_EXCLUSIVE_LOCKS_REQUIRED(mutex_);
|
|
int32_t InitSpeakerLocked() RTC_EXCLUSIVE_LOCKS_REQUIRED(mutex_);
|
|
int32_t InitMicrophoneLocked() RTC_EXCLUSIVE_LOCKS_REQUIRED(mutex_);
|
|
int32_t GetDevicesInfo(const int32_t function,
|
|
const bool playback,
|
|
const int32_t enumDeviceNo = 0,
|
|
char* enumDeviceName = NULL,
|
|
const int32_t ednLen = 0) const;
|
|
int32_t ErrorRecovery(int32_t error, snd_pcm_t* deviceHandle);
|
|
|
|
bool KeyPressed() const;
|
|
|
|
void Lock() RTC_EXCLUSIVE_LOCK_FUNCTION(mutex_) { mutex_.Lock(); }
|
|
void UnLock() RTC_UNLOCK_FUNCTION(mutex_) { mutex_.Unlock(); }
|
|
|
|
inline int32_t InputSanityCheckAfterUnlockedPeriod() const;
|
|
inline int32_t OutputSanityCheckAfterUnlockedPeriod() const;
|
|
|
|
static void RecThreadFunc(void*);
|
|
static void PlayThreadFunc(void*);
|
|
bool RecThreadProcess();
|
|
bool PlayThreadProcess();
|
|
|
|
AudioDeviceBuffer* _ptrAudioBuffer;
|
|
|
|
Mutex mutex_;
|
|
|
|
rtc::PlatformThread _ptrThreadRec;
|
|
rtc::PlatformThread _ptrThreadPlay;
|
|
|
|
AudioMixerManagerLinuxALSA _mixerManager;
|
|
|
|
uint16_t _inputDeviceIndex;
|
|
uint16_t _outputDeviceIndex;
|
|
bool _inputDeviceIsSpecified;
|
|
bool _outputDeviceIsSpecified;
|
|
|
|
snd_pcm_t* _handleRecord;
|
|
snd_pcm_t* _handlePlayout;
|
|
|
|
snd_pcm_uframes_t _recordingBuffersizeInFrame;
|
|
snd_pcm_uframes_t _recordingPeriodSizeInFrame;
|
|
snd_pcm_uframes_t _playoutBufferSizeInFrame;
|
|
snd_pcm_uframes_t _playoutPeriodSizeInFrame;
|
|
|
|
ssize_t _recordingBufferSizeIn10MS;
|
|
ssize_t _playoutBufferSizeIn10MS;
|
|
uint32_t _recordingFramesIn10MS;
|
|
uint32_t _playoutFramesIn10MS;
|
|
|
|
uint32_t _recordingFreq;
|
|
uint32_t _playoutFreq;
|
|
uint8_t _recChannels;
|
|
uint8_t _playChannels;
|
|
|
|
int8_t* _recordingBuffer; // in byte
|
|
int8_t* _playoutBuffer; // in byte
|
|
uint32_t _recordingFramesLeft;
|
|
uint32_t _playoutFramesLeft;
|
|
|
|
bool _initialized;
|
|
bool _recording;
|
|
bool _playing;
|
|
bool _recIsInitialized;
|
|
bool _playIsInitialized;
|
|
|
|
snd_pcm_sframes_t _recordingDelay;
|
|
snd_pcm_sframes_t _playoutDelay;
|
|
|
|
char _oldKeyState[32];
|
|
#if defined(WEBRTC_USE_X11)
|
|
Display* _XDisplay;
|
|
#endif
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_AUDIO_DEVICE_MAIN_SOURCE_LINUX_AUDIO_DEVICE_ALSA_LINUX_H_
|