mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-20 00:57:49 +01:00
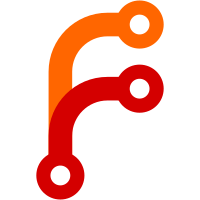
This reverts commit46afbf9481
. Reason for revert: Tightened protocol name handling. Original change's description: > Revert "Reland "Version 2 "Refactoring DataContentDescription class""" > > This reverts commit37f2b43274
. > > Reason for revert: fuzzer failures > > Original change's description: > > Reland "Version 2 "Refactoring DataContentDescription class"" > > > > This is a reland of14b2758726
> > > > Original change's description: > > > Version 2 "Refactoring DataContentDescription class" > > > > > > (substantial changes since version 1) > > > > > > This CL splits the cricket::DataContentDescription class into > > > two classes: cricket::RtpDataContentDescription (used for RTP data) > > > and cricket::SctpDataContentDescription (used for SCTP only). > > > > > > SctpDataContentDescription no longer inherits from > > > MediaContentDescriptionImpl, and no longer contains "codecs". > > > > > > Due to usage of internal interfaces by consumers, shimming the old > > > DataContentDescription API is needed. > > > > > > A new cricket::DataContentDescription class is defined, which is > > > a shim over RtpDataContentDescription and SctpDataContentDescription. > > > It exposes as little functionality as possible, but supports the > > > concerned consumer's usage > > > > > > Design document: > > > https://docs.google.com/document/d/1H5LfQxJA2ikMWTQ8FZ3_GAmaXM7knfVQWiSz6ph8VQ0/edit# > > > > > > Version 1 reviewed-on: https://webrtc-review.googlesource.com/c/src/+/132700 > > > Bug: webrtc:10358 Change-Id: Ia9fb8f4679e082e3d18fbbb6b03fc13a08e06110 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/136581 Reviewed-by: Henrik Boström <hbos@webrtc.org> Reviewed-by: Steve Anton <steveanton@webrtc.org> Commit-Queue: Harald Alvestrand <hta@webrtc.org> Cr-Commit-Position: refs/heads/master@{#27933}
55 lines
1.7 KiB
C++
55 lines
1.7 KiB
C++
/*
|
|
* Copyright 2019 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "pc/media_protocol_names.h"
|
|
|
|
namespace cricket {
|
|
|
|
// There are multiple variants of the RTP protocol stack, including
|
|
// UDP/TLS/RTP/SAVPF (WebRTC default), RTP/AVP, RTP/AVPF, RTP/SAVPF,
|
|
// TCP/DTLS/RTP/SAVPF and so on. We accept anything that has RTP/
|
|
// embedded in it somewhere as being an RTP protocol.
|
|
const char kMediaProtocolRtpPrefix[] = "RTP/";
|
|
|
|
const char kMediaProtocolSctp[] = "SCTP";
|
|
const char kMediaProtocolDtlsSctp[] = "DTLS/SCTP";
|
|
const char kMediaProtocolUdpDtlsSctp[] = "UDP/DTLS/SCTP";
|
|
const char kMediaProtocolTcpDtlsSctp[] = "TCP/DTLS/SCTP";
|
|
|
|
bool IsDtlsSctp(const std::string& protocol) {
|
|
return protocol == kMediaProtocolDtlsSctp ||
|
|
protocol == kMediaProtocolUdpDtlsSctp ||
|
|
protocol == kMediaProtocolTcpDtlsSctp;
|
|
}
|
|
|
|
bool IsPlainSctp(const std::string& protocol) {
|
|
return protocol == kMediaProtocolSctp;
|
|
}
|
|
|
|
bool IsRtpProtocol(const std::string& protocol) {
|
|
if (protocol.empty()) {
|
|
return true;
|
|
}
|
|
size_t pos = protocol.find(cricket::kMediaProtocolRtpPrefix);
|
|
if (pos == std::string::npos) {
|
|
return false;
|
|
}
|
|
// RTP must be at the beginning of a string or not preceded by alpha
|
|
if (pos == 0 || !isalpha(protocol[pos - 1])) {
|
|
return true;
|
|
}
|
|
return false;
|
|
}
|
|
|
|
bool IsSctpProtocol(const std::string& protocol) {
|
|
return IsPlainSctp(protocol) || IsDtlsSctp(protocol);
|
|
}
|
|
|
|
} // namespace cricket
|