mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-18 16:17:50 +01:00
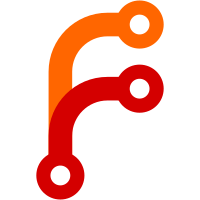
Calculation of quality metrics required writing of decoded video to file. There were two drawbacks with that approach. First, frame drops significantly affected metrics because comparison was done against the last decoded frame. Second, simulcast/SVC required writing of multiple files. This might be too much data to dump. On-fly metrics calculation is done in frame decoded callback. Calculation time is excluded from encoding/decoding time. If CPU usage measurement is enabled metrics calculation is disabled since it affects CPU usage. The results are reported in Stats::PrintSummary. Bug: webrtc:8524 Change-Id: Id54fb21f2f95deeb93757afaf46bde7d7ae18dac Reviewed-on: https://webrtc-review.googlesource.com/22560 Commit-Queue: Sergey Silkin <ssilkin@webrtc.org> Reviewed-by: Patrik Höglund <phoglund@webrtc.org> Reviewed-by: Rasmus Brandt <brandtr@webrtc.org> Cr-Commit-Position: refs/heads/master@{#20798}
83 lines
2.1 KiB
C++
83 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2011 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_VIDEO_CODING_CODECS_TEST_STATS_H_
|
|
#define MODULES_VIDEO_CODING_CODECS_TEST_STATS_H_
|
|
|
|
#include <vector>
|
|
|
|
#include "common_types.h" // NOLINT(build/include)
|
|
|
|
namespace webrtc {
|
|
namespace test {
|
|
|
|
// Statistics for one processed frame.
|
|
struct FrameStatistic {
|
|
explicit FrameStatistic(int frame_number) : frame_number(frame_number) {}
|
|
const int frame_number = 0;
|
|
|
|
// Encoding.
|
|
int64_t encode_start_ns = 0;
|
|
int encode_return_code = 0;
|
|
bool encoding_successful = false;
|
|
int encode_time_us = 0;
|
|
int bitrate_kbps = 0;
|
|
size_t encoded_frame_size_bytes = 0;
|
|
webrtc::FrameType frame_type = kVideoFrameDelta;
|
|
|
|
// H264 specific.
|
|
rtc::Optional<size_t> max_nalu_length;
|
|
|
|
// Decoding.
|
|
int64_t decode_start_ns = 0;
|
|
int decode_return_code = 0;
|
|
bool decoding_successful = false;
|
|
int decode_time_us = 0;
|
|
int decoded_width = 0;
|
|
int decoded_height = 0;
|
|
|
|
// Quantization.
|
|
int qp = -1;
|
|
|
|
// How many packets were discarded of the encoded frame data (if any).
|
|
int packets_dropped = 0;
|
|
size_t total_packets = 0;
|
|
size_t manipulated_length = 0;
|
|
|
|
// Quality.
|
|
float psnr = 0.0;
|
|
float ssim = 0.0;
|
|
};
|
|
|
|
// Statistics for a sequence of processed frames. This class is not thread safe.
|
|
class Stats {
|
|
public:
|
|
Stats() = default;
|
|
~Stats() = default;
|
|
|
|
// Creates a FrameStatistic for the next frame to be processed.
|
|
FrameStatistic* AddFrame();
|
|
|
|
// Returns the FrameStatistic corresponding to |frame_number|.
|
|
FrameStatistic* GetFrame(int frame_number);
|
|
|
|
size_t size() const;
|
|
|
|
// TODO(brandtr): Add output as CSV.
|
|
void PrintSummary() const;
|
|
|
|
private:
|
|
std::vector<FrameStatistic> stats_;
|
|
};
|
|
|
|
} // namespace test
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_VIDEO_CODING_CODECS_TEST_STATS_H_
|