mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
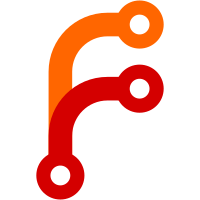
As documented in webrtc:11908 this cleanup is fairly invasive and when a part of a frequently executed code path, can be quite costly in terms of performance overhead. This is currently the case with synchronous calls between threads (Thread) as well with our proxy api classes. With this CL, all code in WebRTC should now either be using MessageHandlerAutoCleanup or calling MessageHandler(false) explicitly. Next steps will be to update external code to either depend on the AutoCleanup variant, or call MessageHandler(false). Changing the proxy classes to use TaskQueue set of concepts instead of MessageHandler. This avoids the perf overhead related to the cleanup above as well as incompatibility with the thread policy checks in Thread that some current external users of the proxies would otherwise run into (if we were to use Thread::Send() for synchronous call). Following this we'll move the cleanup step into the AutoCleanup class and an RTC_DCHECK that all calls to the MessageHandler are setting the flag to false, before eventually removing the flag and make MessageHandler pure virtual. Bug: webrtc:11908 Change-Id: Idf4ff9bcc8438cb8c583777e282005e0bc511c8f Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/183442 Reviewed-by: Artem Titov <titovartem@webrtc.org> Commit-Queue: Tommi <tommi@webrtc.org> Cr-Commit-Position: refs/heads/master@{#32049}
53 lines
1.5 KiB
C++
53 lines
1.5 KiB
C++
/*
|
|
* Copyright 2012 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "rtc_base/null_socket_server.h"
|
|
|
|
#include <stdint.h>
|
|
|
|
#include <memory>
|
|
|
|
#include "rtc_base/gunit.h"
|
|
#include "rtc_base/location.h"
|
|
#include "rtc_base/message_handler.h"
|
|
#include "rtc_base/thread.h"
|
|
#include "rtc_base/time_utils.h"
|
|
#include "test/gtest.h"
|
|
|
|
namespace rtc {
|
|
|
|
static const uint32_t kTimeout = 5000U;
|
|
|
|
class NullSocketServerTest : public ::testing::Test,
|
|
public MessageHandlerAutoCleanup {
|
|
protected:
|
|
void OnMessage(Message* message) override { ss_.WakeUp(); }
|
|
|
|
NullSocketServer ss_;
|
|
};
|
|
|
|
TEST_F(NullSocketServerTest, WaitAndSet) {
|
|
auto thread = Thread::Create();
|
|
EXPECT_TRUE(thread->Start());
|
|
thread->Post(RTC_FROM_HERE, this, 0);
|
|
// The process_io will be ignored.
|
|
const bool process_io = true;
|
|
EXPECT_TRUE_WAIT(ss_.Wait(SocketServer::kForever, process_io), kTimeout);
|
|
}
|
|
|
|
TEST_F(NullSocketServerTest, TestWait) {
|
|
int64_t start = TimeMillis();
|
|
ss_.Wait(200, true);
|
|
// The actual wait time is dependent on the resolution of the timer used by
|
|
// the Event class. Allow for the event to signal ~20ms early.
|
|
EXPECT_GE(TimeSince(start), 180);
|
|
}
|
|
|
|
} // namespace rtc
|