mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
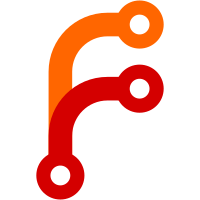
Done in preparation for some threading changes that would be quite messy if implemented with the class as-is. This results in some code duplication, but is preferable to one class having two completely different modes of operation. RTP data channels are in the process of being removed anyway, so the duplicated code won't last forever. Bug: webrtc:9883 Change-Id: Idfd41a669b56a4bb4819572e4a264a4ffaaba9c0 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/178940 Commit-Queue: Taylor <deadbeef@webrtc.org> Reviewed-by: Harald Alvestrand <hta@webrtc.org> Cr-Commit-Position: refs/heads/master@{#31691}
56 lines
1.6 KiB
C++
56 lines
1.6 KiB
C++
/*
|
|
* Copyright 2020 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "pc/data_channel_utils.h"
|
|
|
|
namespace webrtc {
|
|
|
|
bool PacketQueue::Empty() const {
|
|
return packets_.empty();
|
|
}
|
|
|
|
std::unique_ptr<DataBuffer> PacketQueue::PopFront() {
|
|
RTC_DCHECK(!packets_.empty());
|
|
byte_count_ -= packets_.front()->size();
|
|
std::unique_ptr<DataBuffer> packet = std::move(packets_.front());
|
|
packets_.pop_front();
|
|
return packet;
|
|
}
|
|
|
|
void PacketQueue::PushFront(std::unique_ptr<DataBuffer> packet) {
|
|
byte_count_ += packet->size();
|
|
packets_.push_front(std::move(packet));
|
|
}
|
|
|
|
void PacketQueue::PushBack(std::unique_ptr<DataBuffer> packet) {
|
|
byte_count_ += packet->size();
|
|
packets_.push_back(std::move(packet));
|
|
}
|
|
|
|
void PacketQueue::Clear() {
|
|
packets_.clear();
|
|
byte_count_ = 0;
|
|
}
|
|
|
|
void PacketQueue::Swap(PacketQueue* other) {
|
|
size_t other_byte_count = other->byte_count_;
|
|
other->byte_count_ = byte_count_;
|
|
byte_count_ = other_byte_count;
|
|
|
|
other->packets_.swap(packets_);
|
|
}
|
|
|
|
bool IsSctpLike(cricket::DataChannelType type) {
|
|
return type == cricket::DCT_SCTP || type == cricket::DCT_MEDIA_TRANSPORT ||
|
|
type == cricket::DCT_DATA_CHANNEL_TRANSPORT ||
|
|
type == cricket::DCT_DATA_CHANNEL_TRANSPORT_SCTP;
|
|
}
|
|
|
|
} // namespace webrtc
|