mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
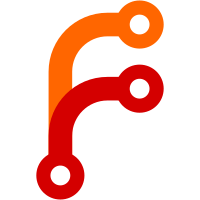
One reason for the circular deps is that common_types.h is a historical dumping ground for various structs and defines that are believed to be generally useful. I tried moving things out that did not appear to be used downstream (StreamCounters, RtpCounters etc) and moved the things that seemed used (RtpHeader + supporting structs) to a new file api/rtp_headers.h. This makes their place in the api more clear while moving out the things that don't belong in the API in the first place. I had to extract out typedefs.h from webrtc_common to resolve another circular dependency. I believe checks includes typedefs, but common depends on checks. Bug: webrtc:7745 Change-Id: I725d49616b1ec0cdc8b74be7c078f7a4d46f084b Reviewed-on: https://webrtc-review.googlesource.com/33001 Commit-Queue: Patrik Höglund <phoglund@webrtc.org> Reviewed-by: Karl Wiberg <kwiberg@webrtc.org> Cr-Commit-Position: refs/heads/master@{#21295}
64 lines
2 KiB
C++
64 lines
2 KiB
C++
/*
|
|
* Copyright (c) 2015 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#ifndef SYSTEM_WRAPPERS_INCLUDE_NTP_TIME_H_
|
|
#define SYSTEM_WRAPPERS_INCLUDE_NTP_TIME_H_
|
|
|
|
#include <stdint.h>
|
|
|
|
#include "rtc_base/numerics/safe_conversions.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class NtpTime {
|
|
public:
|
|
static constexpr uint64_t kFractionsPerSecond = 0x100000000;
|
|
NtpTime() : value_(0) {}
|
|
explicit NtpTime(uint64_t value) : value_(value) {}
|
|
NtpTime(uint32_t seconds, uint32_t fractions)
|
|
: value_(seconds * kFractionsPerSecond + fractions) {}
|
|
|
|
NtpTime(const NtpTime&) = default;
|
|
NtpTime& operator=(const NtpTime&) = default;
|
|
explicit operator uint64_t() const { return value_; }
|
|
|
|
void Set(uint32_t seconds, uint32_t fractions) {
|
|
value_ = seconds * kFractionsPerSecond + fractions;
|
|
}
|
|
void Reset() { value_ = 0; }
|
|
|
|
int64_t ToMs() const {
|
|
static constexpr double kNtpFracPerMs = 4.294967296E6; // 2^32 / 1000.
|
|
const double frac_ms = static_cast<double>(fractions()) / kNtpFracPerMs;
|
|
return 1000 * static_cast<int64_t>(seconds()) +
|
|
static_cast<int64_t>(frac_ms + 0.5);
|
|
}
|
|
// NTP standard (RFC1305, section 3.1) explicitly state value 0 is invalid.
|
|
bool Valid() const { return value_ != 0; }
|
|
|
|
uint32_t seconds() const {
|
|
return rtc::dchecked_cast<uint32_t>(value_ / kFractionsPerSecond);
|
|
}
|
|
uint32_t fractions() const {
|
|
return rtc::dchecked_cast<uint32_t>(value_ % kFractionsPerSecond);
|
|
}
|
|
|
|
private:
|
|
uint64_t value_;
|
|
};
|
|
|
|
inline bool operator==(const NtpTime& n1, const NtpTime& n2) {
|
|
return static_cast<uint64_t>(n1) == static_cast<uint64_t>(n2);
|
|
}
|
|
inline bool operator!=(const NtpTime& n1, const NtpTime& n2) {
|
|
return !(n1 == n2);
|
|
}
|
|
|
|
} // namespace webrtc
|
|
#endif // SYSTEM_WRAPPERS_INCLUDE_NTP_TIME_H_
|