mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 22:00:47 +01:00
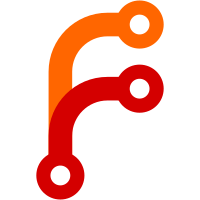
This change introduces a clockdrift detector operating on the estimated delay of the echo path delay estimator. Each time the delay estimate changes it is compared to previous estimates. If the estimates are slowly increasing or decreasing, clockdrift is detected. Four different patterns are considered clockdrift: - k, k+1, k+2, k+3 - k, k+2, k+1, k+3 - k, k-1, k-2, k-3 - k, k-2, k-1, k-3 A delay estimate history matching the three last elements in one of the patterns is considered probable clockdrift. Matching all four elements is considered verified clockdrift. If the delay is constant for some time after clockdrift is detected the clockdrift detector will revert to no detected clockdrift. The level of clockdrift is reported via an UMA histogram. Bug: webrtc:10014 Change-Id: I1cce4d593e101a8b3fa99df6935e59b4243cb97a Reviewed-on: https://webrtc-review.googlesource.com/c/111381 Commit-Queue: Gustaf Ullberg <gustaf@webrtc.org> Reviewed-by: Per Åhgren <peah@webrtc.org> Cr-Commit-Position: refs/heads/master@{#25758}
61 lines
2 KiB
C++
61 lines
2 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#include "modules/audio_processing/aec3/clockdrift_detector.h"
|
|
|
|
namespace webrtc {
|
|
|
|
ClockdriftDetector::ClockdriftDetector()
|
|
: level_(Level::kNone), stability_counter_(0) {
|
|
delay_history_.fill(0);
|
|
}
|
|
|
|
ClockdriftDetector::~ClockdriftDetector() = default;
|
|
|
|
void ClockdriftDetector::Update(int delay_estimate) {
|
|
if (delay_estimate == delay_history_[0]) {
|
|
// Reset clockdrift level if delay estimate is stable for 7500 blocks (30
|
|
// seconds).
|
|
if (++stability_counter_ > 7500)
|
|
level_ = Level::kNone;
|
|
return;
|
|
}
|
|
|
|
stability_counter_ = 0;
|
|
const int d1 = delay_history_[0] - delay_estimate;
|
|
const int d2 = delay_history_[1] - delay_estimate;
|
|
const int d3 = delay_history_[2] - delay_estimate;
|
|
|
|
// Patterns recognized as positive clockdrift:
|
|
// [x-3], x-2, x-1, x.
|
|
// [x-3], x-1, x-2, x.
|
|
const bool probable_drift_up =
|
|
(d1 == -1 && d2 == -2) || (d1 == -2 && d2 == -1);
|
|
const bool drift_up = probable_drift_up && d3 == -3;
|
|
|
|
// Patterns recognized as negative clockdrift:
|
|
// [x+3], x+2, x+1, x.
|
|
// [x+3], x+1, x+2, x.
|
|
const bool probable_drift_down = (d1 == 1 && d2 == 2) || (d1 == 2 && d2 == 1);
|
|
const bool drift_down = probable_drift_down && d3 == 3;
|
|
|
|
// Set clockdrift level.
|
|
if (drift_up || drift_down) {
|
|
level_ = Level::kVerified;
|
|
} else if ((probable_drift_up || probable_drift_down) &&
|
|
level_ == Level::kNone) {
|
|
level_ = Level::kProbable;
|
|
}
|
|
|
|
// Shift delay history one step.
|
|
delay_history_[2] = delay_history_[1];
|
|
delay_history_[1] = delay_history_[0];
|
|
delay_history_[0] = delay_estimate;
|
|
}
|
|
} // namespace webrtc
|