mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-16 07:10:38 +01:00
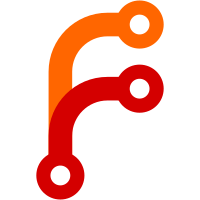
This adds the ability to the pacer to apply a congestion window by tracking sent data. This makes it more reliable when the congestion window is small enough to be filled at a high rate as there are less thread context switches that might affect the timing and performance. Outstanding data is not reduced by the pacer as it has no information about acknowledged packet feedback. This is by design as the pacer would also need to keep track of on which connection packets were sent or received, requiring a larger, more complex, change to the pacer. Bug: webrtc:8415 Change-Id: I4ecd303e835552ced042cd21186da910288a8258 Reviewed-on: https://webrtc-review.googlesource.com/51764 Reviewed-by: Philip Eliasson <philipel@webrtc.org> Commit-Queue: Sebastian Jansson <srte@webrtc.org> Cr-Commit-Position: refs/heads/master@{#22371}
53 lines
1.8 KiB
C++
53 lines
1.8 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_CONGESTION_CONTROLLER_RTP_PACER_CONTROLLER_H_
|
|
#define MODULES_CONGESTION_CONTROLLER_RTP_PACER_CONTROLLER_H_
|
|
|
|
#include <memory>
|
|
|
|
#include "modules/congestion_controller/network_control/include/network_types.h"
|
|
#include "modules/pacing/paced_sender.h"
|
|
#include "rtc_base/sequenced_task_checker.h"
|
|
|
|
namespace webrtc {
|
|
class Clock;
|
|
|
|
namespace webrtc_cc {
|
|
|
|
// Wrapper class to control pacer using task queues. Note that this class is
|
|
// only designed to be used from a single task queue and has no built in
|
|
// concurrency safety.
|
|
// TODO(srte): Integrate this interface directly into PacedSender.
|
|
class PacerController {
|
|
public:
|
|
explicit PacerController(PacedSender* pacer);
|
|
~PacerController();
|
|
void OnCongestionWindow(CongestionWindow msg);
|
|
void OnNetworkAvailability(NetworkAvailability msg);
|
|
void OnNetworkRouteChange(NetworkRouteChange msg);
|
|
void OnOutstandingData(OutstandingData msg);
|
|
void OnPacerConfig(PacerConfig msg);
|
|
void OnProbeClusterConfig(ProbeClusterConfig msg);
|
|
|
|
private:
|
|
void SetPacerState(bool paused);
|
|
PacedSender* const pacer_;
|
|
|
|
rtc::Optional<PacerConfig> current_pacer_config_;
|
|
bool pacer_paused_ = false;
|
|
bool network_available_ = true;
|
|
|
|
rtc::SequencedTaskChecker sequenced_checker_;
|
|
RTC_DISALLOW_IMPLICIT_CONSTRUCTORS(PacerController);
|
|
};
|
|
} // namespace webrtc_cc
|
|
} // namespace webrtc
|
|
#endif // MODULES_CONGESTION_CONTROLLER_RTP_PACER_CONTROLLER_H_
|