mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-19 00:27:51 +01:00
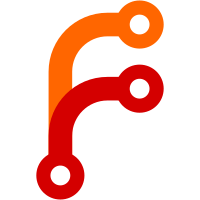
This required a fairly extensive overhaul: * Removed locks from the implementation. The filter/pin architecture does use multiple threads, but the state is controlled on one and synchronization is done via flags that don't require locking. Note though that the baseclasses used a lot of locking, it's unclear why, but perhaps there are things I'm not aware of. The locking was not done consistently though, which doesn't seem to have been a problem. * Change the code to not mix AddRef/Release and use of explicit 'delete'. * Removed implementations of interfaces we don't need/use. * Similarly some methods now return E_NOTIMPL. * Added some utilities to make use of COM interfaces and concepts, easier. BUG=webrtc:10374 TBR=mbonadei@webrtc.org Change-Id: Iaedb1157d37ef5d5c75f727dba3d7de75ce22cd8 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/125086 Commit-Queue: Tommi <tommi@webrtc.org> Reviewed-by: Christian Fremerey <chfremer@webrtc.org> Cr-Commit-Position: refs/heads/master@{#27072}
74 lines
2.3 KiB
C++
74 lines
2.3 KiB
C++
/*
|
|
* Copyright (c) 2012 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_VIDEO_CAPTURE_MAIN_SOURCE_WINDOWS_VIDEO_CAPTURE_DS_H_
|
|
#define MODULES_VIDEO_CAPTURE_MAIN_SOURCE_WINDOWS_VIDEO_CAPTURE_DS_H_
|
|
|
|
#include "api/scoped_refptr.h"
|
|
#include "modules/video_capture/video_capture_impl.h"
|
|
#include "modules/video_capture/windows/device_info_ds.h"
|
|
|
|
#define CAPTURE_FILTER_NAME L"VideoCaptureFilter"
|
|
#define SINK_FILTER_NAME L"SinkFilter"
|
|
|
|
namespace webrtc {
|
|
namespace videocapturemodule {
|
|
// Forward declaraion
|
|
class CaptureSinkFilter;
|
|
|
|
class VideoCaptureDS : public VideoCaptureImpl {
|
|
public:
|
|
VideoCaptureDS();
|
|
|
|
virtual int32_t Init(const char* deviceUniqueIdUTF8);
|
|
|
|
/*************************************************************************
|
|
*
|
|
* Start/Stop
|
|
*
|
|
*************************************************************************/
|
|
int32_t StartCapture(const VideoCaptureCapability& capability) override;
|
|
int32_t StopCapture() override;
|
|
|
|
/**************************************************************************
|
|
*
|
|
* Properties of the set device
|
|
*
|
|
**************************************************************************/
|
|
|
|
bool CaptureStarted() override;
|
|
int32_t CaptureSettings(VideoCaptureCapability& settings) override;
|
|
|
|
protected:
|
|
~VideoCaptureDS() override;
|
|
|
|
// Help functions
|
|
|
|
int32_t SetCameraOutput(const VideoCaptureCapability& requestedCapability);
|
|
int32_t DisconnectGraph();
|
|
HRESULT ConnectDVCamera();
|
|
|
|
DeviceInfoDS _dsInfo;
|
|
|
|
IBaseFilter* _captureFilter;
|
|
IGraphBuilder* _graphBuilder;
|
|
IMediaControl* _mediaControl;
|
|
rtc::scoped_refptr<CaptureSinkFilter> sink_filter_;
|
|
IPin* _inputSendPin;
|
|
IPin* _outputCapturePin;
|
|
|
|
// Microsoft DV interface (external DV cameras)
|
|
IBaseFilter* _dvFilter;
|
|
IPin* _inputDvPin;
|
|
IPin* _outputDvPin;
|
|
};
|
|
} // namespace videocapturemodule
|
|
} // namespace webrtc
|
|
#endif // MODULES_VIDEO_CAPTURE_MAIN_SOURCE_WINDOWS_VIDEO_CAPTURE_DS_H_
|