mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
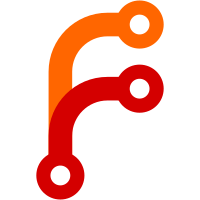
For implementations where the signaling and worker threads are not the same thread, this significantly cuts down on Thread::Invoke()s that would block the signaling thread while waiting for the worker thread. For Audio and Video Rtp receivers, the following methods now do not block the signaling thread: * GetParameters * SetJitterBufferMinimumDelay * GetSources * SetFrameDecryptor / GetFrameDecryptor * SetDepacketizerToDecoderFrameTransformer Importantly this change also makes the track() accessor accessible directly from the application thread (bypassing the proxy) since for receiver objects, the track object is const. Other changes: * Remove RefCountedObject inheritance, use make_ref_counted instead. * Every member variable in the rtp receiver classes is now RTC_GUARDED * Stop() now fully clears up worker thread state, and Stop() is consistently called before destruction. This means that there's one thread hop instead of at least 4 before (sometimes more), per receiver. * OnChanged triggered volume for audio tracks is done asynchronously. * Deleted most of the JitterBufferDelay implementation. Turns out that it was largely unnecessary overhead and complexity. It seems that these two classes are copy/pasted to a large extent so further refactoring would be good in the future, as to not have to fix each issue twice. Bug: chromium:1184611 Change-Id: I1ba5c3abbd1b0571f7d12850d64004fd2d83e5e2 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/218605 Commit-Queue: Tommi <tommi@webrtc.org> Reviewed-by: Markus Handell <handellm@webrtc.org> Cr-Commit-Position: refs/heads/master@{#34022}
40 lines
1.2 KiB
C++
40 lines
1.2 KiB
C++
/*
|
|
* Copyright 2019 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef PC_JITTER_BUFFER_DELAY_H_
|
|
#define PC_JITTER_BUFFER_DELAY_H_
|
|
|
|
#include <stdint.h>
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "api/sequence_checker.h"
|
|
#include "rtc_base/system/no_unique_address.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// JitterBufferDelay converts delay from seconds to milliseconds for the
|
|
// underlying media channel. It also handles cases when user sets delay before
|
|
// the start of media_channel by caching its request.
|
|
class JitterBufferDelay {
|
|
public:
|
|
JitterBufferDelay();
|
|
|
|
void Set(absl::optional<double> delay_seconds);
|
|
int GetMs() const;
|
|
|
|
private:
|
|
RTC_NO_UNIQUE_ADDRESS SequenceChecker worker_thread_checker_;
|
|
absl::optional<double> cached_delay_seconds_
|
|
RTC_GUARDED_BY(&worker_thread_checker_);
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // PC_JITTER_BUFFER_DELAY_H_
|