mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
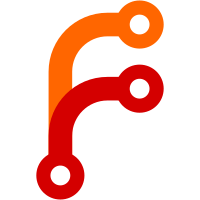
For implementations where the signaling and worker threads are not the same thread, this significantly cuts down on Thread::Invoke()s that would block the signaling thread while waiting for the worker thread. For Audio and Video Rtp receivers, the following methods now do not block the signaling thread: * GetParameters * SetJitterBufferMinimumDelay * GetSources * SetFrameDecryptor / GetFrameDecryptor * SetDepacketizerToDecoderFrameTransformer Importantly this change also makes the track() accessor accessible directly from the application thread (bypassing the proxy) since for receiver objects, the track object is const. Other changes: * Remove RefCountedObject inheritance, use make_ref_counted instead. * Every member variable in the rtp receiver classes is now RTC_GUARDED * Stop() now fully clears up worker thread state, and Stop() is consistently called before destruction. This means that there's one thread hop instead of at least 4 before (sometimes more), per receiver. * OnChanged triggered volume for audio tracks is done asynchronously. * Deleted most of the JitterBufferDelay implementation. Turns out that it was largely unnecessary overhead and complexity. It seems that these two classes are copy/pasted to a large extent so further refactoring would be good in the future, as to not have to fix each issue twice. Bug: chromium:1184611 Change-Id: I1ba5c3abbd1b0571f7d12850d64004fd2d83e5e2 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/218605 Commit-Queue: Tommi <tommi@webrtc.org> Reviewed-by: Markus Handell <handellm@webrtc.org> Cr-Commit-Position: refs/heads/master@{#34022}
59 lines
1.4 KiB
C++
59 lines
1.4 KiB
C++
/*
|
|
* Copyright 2019 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "pc/jitter_buffer_delay.h"
|
|
|
|
#include <stdint.h>
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "test/gtest.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class JitterBufferDelayTest : public ::testing::Test {
|
|
public:
|
|
JitterBufferDelayTest() {}
|
|
|
|
protected:
|
|
JitterBufferDelay delay_;
|
|
};
|
|
|
|
TEST_F(JitterBufferDelayTest, Set) {
|
|
// Delay in seconds.
|
|
delay_.Set(3.0);
|
|
EXPECT_EQ(delay_.GetMs(), 3000);
|
|
}
|
|
|
|
TEST_F(JitterBufferDelayTest, DefaultValue) {
|
|
EXPECT_EQ(delay_.GetMs(), 0); // Default value is 0ms.
|
|
}
|
|
|
|
TEST_F(JitterBufferDelayTest, Clamping) {
|
|
// In current Jitter Buffer implementation (Audio or Video) maximum supported
|
|
// value is 10000 milliseconds.
|
|
delay_.Set(10.5);
|
|
EXPECT_EQ(delay_.GetMs(), 10000);
|
|
|
|
// Test int overflow.
|
|
delay_.Set(21474836470.0);
|
|
EXPECT_EQ(delay_.GetMs(), 10000);
|
|
|
|
delay_.Set(-21474836470.0);
|
|
EXPECT_EQ(delay_.GetMs(), 0);
|
|
|
|
// Boundary value in seconds to milliseconds conversion.
|
|
delay_.Set(0.0009);
|
|
EXPECT_EQ(delay_.GetMs(), 0);
|
|
|
|
delay_.Set(-2.0);
|
|
EXPECT_EQ(delay_.GetMs(), 0);
|
|
}
|
|
|
|
} // namespace webrtc
|