mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 14:20:45 +01:00
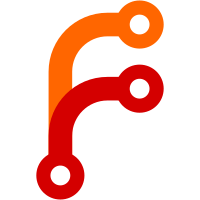
This change introduces a new class BufferedFrameDecryptor that is responsible for decrypting received encrypted frames and passing them on to the RtpReferenceFinder. This decoupling refactoring was triggered by a new optimization also introduced in this patch to stash a small number of undecryptable frames if no frames have ever been decrypted. The goal of this optimization is to prevent re-fectching of key frames on low bandwidth networks simply because the key to decrypt them had not arrived yet. The optimization will stash 24 frames (about 1 second of video) in a ring buffer and will attempt to re-decrypt previously received frames on the first valid decryption. This allows the decoder to receive the key frame without having to request due to short key delivery latencies. In testing this is actually hit quite often and saves an entire RTT which can be up to 200ms on a bad network. As the scope of frame encryption increases in WebRTC and has more specialized optimizations that do not apply to the general flow it makes sense to move it to a more explicit bump in the stack protocol that is decoupled from the WebRTC main flow, similar to how SRTP is utilized with srtp_protect and srtp_unprotect. One advantage of this approach is the BufferedFrameDecryptor isn't even constructed if FrameEncryption is not in use. I have decided against merging the RtpReferenceFinder and EncryptedFrame stash because it introduced a lot of complexity around the mixed scenario where some of the frames in the stash are encrypted and others are not. In this case we would need to mark certain frames as decrypted which appeared to introduce more complexity than this simple decoupling. Bug: webrtc:10022 Change-Id: Iab74f7b7d25ef1cdd15c4a76b5daae1cfa24932c Reviewed-on: https://webrtc-review.googlesource.com/c/112221 Commit-Queue: Benjamin Wright <benwright@webrtc.org> Reviewed-by: Philip Eliasson <philipel@webrtc.org> Reviewed-by: Stefan Holmer <stefan@webrtc.org> Cr-Commit-Position: refs/heads/master@{#25865}
66 lines
2.1 KiB
C++
66 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_VIDEO_CODING_FRAME_OBJECT_H_
|
|
#define MODULES_VIDEO_CODING_FRAME_OBJECT_H_
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "api/video/encoded_frame.h"
|
|
#include "common_types.h" // NOLINT(build/include)
|
|
#include "modules/include/module_common_types.h"
|
|
#include "modules/rtp_rtcp/source/rtp_generic_frame_descriptor.h"
|
|
|
|
namespace webrtc {
|
|
namespace video_coding {
|
|
|
|
class PacketBuffer;
|
|
|
|
class RtpFrameObject : public EncodedFrame {
|
|
public:
|
|
RtpFrameObject(PacketBuffer* packet_buffer,
|
|
uint16_t first_seq_num,
|
|
uint16_t last_seq_num,
|
|
size_t frame_size,
|
|
int times_nacked,
|
|
int64_t received_time);
|
|
|
|
~RtpFrameObject() override;
|
|
uint16_t first_seq_num() const;
|
|
uint16_t last_seq_num() const;
|
|
int times_nacked() const;
|
|
enum FrameType frame_type() const;
|
|
VideoCodecType codec_type() const;
|
|
int64_t ReceivedTime() const override;
|
|
int64_t RenderTime() const override;
|
|
void SetSize(size_t size);
|
|
bool delayed_by_retransmission() const override;
|
|
absl::optional<RTPVideoHeader> GetRtpVideoHeader() const;
|
|
absl::optional<RtpGenericFrameDescriptor> GetGenericFrameDescriptor() const;
|
|
absl::optional<FrameMarking> GetFrameMarking() const;
|
|
|
|
private:
|
|
void AllocateBitstreamBuffer(size_t frame_size);
|
|
|
|
rtc::scoped_refptr<PacketBuffer> packet_buffer_;
|
|
enum FrameType frame_type_;
|
|
VideoCodecType codec_type_;
|
|
uint16_t first_seq_num_;
|
|
uint16_t last_seq_num_;
|
|
int64_t received_time_;
|
|
|
|
// Equal to times nacked of the packet with the highet times nacked
|
|
// belonging to this frame.
|
|
int times_nacked_;
|
|
};
|
|
|
|
} // namespace video_coding
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_VIDEO_CODING_FRAME_OBJECT_H_
|