mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 22:00:47 +01:00
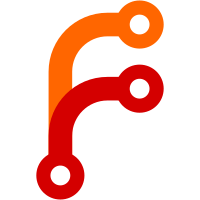
Add ability to provide custom implementation of NetworkSimulatedInterface for sender and receiver network in VideoQualityTestFixtureInterface, passing them to the factory method. Also unite this mechanism with FecControllerFactoryInterface injection. Bug: webrtc:9630 Change-Id: I79259113e0fc00d933b73ca299afa836a4cd19d2 Reviewed-on: https://webrtc-review.googlesource.com/96280 Commit-Queue: Artem Titov <titovartem@webrtc.org> Reviewed-by: Patrik Höglund <phoglund@webrtc.org> Reviewed-by: Erik Språng <sprang@webrtc.org> Cr-Commit-Position: refs/heads/master@{#24476}
43 lines
1.5 KiB
C++
43 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include <memory>
|
|
#include <utility>
|
|
|
|
#include "absl/memory/memory.h"
|
|
#include "api/test/create_video_quality_test_fixture.h"
|
|
#include "video/video_quality_test.h"
|
|
|
|
namespace webrtc {
|
|
|
|
std::unique_ptr<VideoQualityTestFixtureInterface>
|
|
CreateVideoQualityTestFixture() {
|
|
// By default, we don't override the FEC module, so pass an empty factory.
|
|
return absl::make_unique<VideoQualityTest>(nullptr);
|
|
}
|
|
|
|
std::unique_ptr<VideoQualityTestFixtureInterface>
|
|
CreateVideoQualityTestFixture(
|
|
std::unique_ptr<FecControllerFactoryInterface> fec_controller_factory) {
|
|
auto components = absl::make_unique<
|
|
VideoQualityTestFixtureInterface::InjectionComponents>();
|
|
components->fec_controller_factory = std::move(fec_controller_factory);
|
|
return absl::make_unique<VideoQualityTest>(std::move(components));
|
|
}
|
|
|
|
std::unique_ptr<VideoQualityTestFixtureInterface> CreateVideoQualityTestFixture(
|
|
std::unique_ptr<VideoQualityTestFixtureInterface::InjectionComponents>
|
|
components) {
|
|
return absl::make_unique<VideoQualityTest>(std::move(components));
|
|
}
|
|
|
|
} // namespace webrtc
|
|
|
|
|