mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
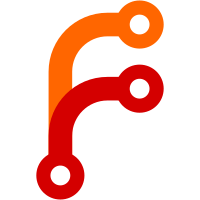
https://chromium.googlesource.com/chromium/src/+/master/styleguide/inclusive_code.md#tools Not changin the transcipt in resources/audio_processing/conversational_speech/README.md BUG=webrtc:11680 Change-Id: I36af34e4a4e0ec6161093c0045b7bbe1dbe4eb45 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/177016 Reviewed-by: Tommi <tommi@webrtc.org> Cr-Commit-Position: refs/heads/master@{#31514}
53 lines
2.2 KiB
C++
53 lines
2.2 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef LOGGING_RTC_EVENT_LOG_ENCODER_BLOB_ENCODING_H_
|
|
#define LOGGING_RTC_EVENT_LOG_ENCODER_BLOB_ENCODING_H_
|
|
|
|
#include <stddef.h>
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "absl/strings/string_view.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// Encode/decode a sequence of strings, whose length is not known to be
|
|
// discernable from the blob itself (i.e. without being transmitted OOB),
|
|
// in a way that would allow us to separate them again on the decoding side.
|
|
// The number of blobs is assumed to be transmitted OOB. For example, if
|
|
// multiple sequences of different blobs are sent, but all sequences contain
|
|
// the same number of blobs, it is beneficial to not encode the number of blobs.
|
|
//
|
|
// EncodeBlobs() must be given a non-empty vector. The blobs themselves may
|
|
// be equal to "", though.
|
|
// EncodeBlobs() may not fail.
|
|
// EncodeBlobs() never returns the empty string.
|
|
//
|
|
// Calling DecodeBlobs() on an empty string, or with |num_of_blobs| set to 0,
|
|
// is an error.
|
|
// DecodeBlobs() returns an empty vector if it fails, e.g. due to a mismatch
|
|
// between |num_of_blobs| and |encoded_blobs|, which can happen if
|
|
// |encoded_blobs| is corrupted.
|
|
// When successful, DecodeBlobs() returns a vector of string_view objects,
|
|
// which refer to the original input (|encoded_blobs|), and therefore may
|
|
// not outlive it.
|
|
//
|
|
// Note that the returned std::string might have been reserved for significantly
|
|
// more memory than it ends up using. If the caller to EncodeBlobs() intends
|
|
// to store the result long-term, they should consider shrink_to_fit()-ing it.
|
|
std::string EncodeBlobs(const std::vector<std::string>& blobs);
|
|
std::vector<absl::string_view> DecodeBlobs(absl::string_view encoded_blobs,
|
|
size_t num_of_blobs);
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // LOGGING_RTC_EVENT_LOG_ENCODER_BLOB_ENCODING_H_
|