mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 14:20:45 +01:00
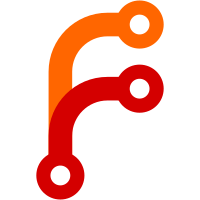
PostDelayedTask doesn't guarantee task execution order. For example, if you post two tasks, A and B, back-to-back using the same delay there is no guarantee that A will be executed before B. Re-implemented pacing using sleep(). Changed pacer to compute task scheduled time instead of delay. Sleep time is calculated right before task start. This provides better accuracy by accounting for any delays that may happen after pacing time is computed and before task queue is ready to run the task. It is tricky to implement pacer tests using simulated clocks. The test use system time which make them flacky on low performance bots. Keep the test disabled by default. Bug: b/261160916, webrtc:14852 Change-Id: I88e1a2001e6d33cf3bb7fe16730ec28abf90acc8 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/291804 Reviewed-by: Rasmus Brandt <brandtr@webrtc.org> Commit-Queue: Sergey Silkin <ssilkin@webrtc.org> Cr-Commit-Position: refs/heads/main@{#39302}
45 lines
1.5 KiB
C++
45 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2022 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_VIDEO_CODING_CODECS_TEST_VIDEO_CODEC_TESTER_IMPL_H_
|
|
#define MODULES_VIDEO_CODING_CODECS_TEST_VIDEO_CODEC_TESTER_IMPL_H_
|
|
|
|
#include <memory>
|
|
|
|
#include "api/test/video_codec_tester.h"
|
|
|
|
namespace webrtc {
|
|
namespace test {
|
|
|
|
// A stateless implementation of `VideoCodecTester`. This class is thread safe.
|
|
class VideoCodecTesterImpl : public VideoCodecTester {
|
|
public:
|
|
std::unique_ptr<VideoCodecStats> RunDecodeTest(
|
|
CodedVideoSource* video_source,
|
|
Decoder* decoder,
|
|
const DecoderSettings& decoder_settings) override;
|
|
|
|
std::unique_ptr<VideoCodecStats> RunEncodeTest(
|
|
RawVideoSource* video_source,
|
|
Encoder* encoder,
|
|
const EncoderSettings& encoder_settings) override;
|
|
|
|
std::unique_ptr<VideoCodecStats> RunEncodeDecodeTest(
|
|
RawVideoSource* video_source,
|
|
Encoder* encoder,
|
|
Decoder* decoder,
|
|
const EncoderSettings& encoder_settings,
|
|
const DecoderSettings& decoder_settings) override;
|
|
};
|
|
|
|
} // namespace test
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_VIDEO_CODING_CODECS_TEST_VIDEO_CODEC_TESTER_IMPL_H_
|