mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
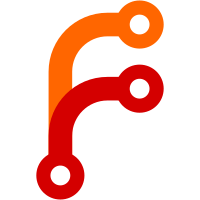
This renames the tests to also capture the expected outcome of the test along with some minor code cleanups. Some tests have also been added or extended to tests more invariants. Bug: None Change-Id: I0bc733026118eb90646929b164bfc148665556a8 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/267169 Reviewed-by: Jonas Oreland <jonaso@webrtc.org> Commit-Queue: Per Kjellander <perkj@webrtc.org> Reviewed-by: Per Kjellander <perkj@webrtc.org> Auto-Submit: Emil Lundmark <lndmrk@webrtc.org> Cr-Commit-Position: refs/heads/main@{#37673}
128 lines
4.3 KiB
C++
128 lines
4.3 KiB
C++
/*
|
|
* Copyright 2022 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "api/field_trials.h"
|
|
|
|
#include <memory>
|
|
|
|
#include "api/transport/field_trial_based_config.h"
|
|
#include "system_wrappers/include/field_trial.h"
|
|
#include "test/gmock.h"
|
|
#include "test/gtest.h"
|
|
|
|
#if GTEST_HAS_DEATH_TEST && !defined(WEBRTC_ANDROID)
|
|
#include "test/testsupport/rtc_expect_death.h"
|
|
#endif // GTEST_HAS_DEATH_TEST && !defined(WEBRTC_ANDROID)
|
|
|
|
namespace webrtc {
|
|
namespace {
|
|
|
|
using ::testing::NotNull;
|
|
|
|
TEST(FieldTrialsTest, EmptyStringHasNoEffect) {
|
|
FieldTrials f("");
|
|
EXPECT_FALSE(f.IsEnabled("MyCoolTrial"));
|
|
EXPECT_FALSE(f.IsDisabled("MyCoolTrial"));
|
|
}
|
|
|
|
TEST(FieldTrialsTest, EnabledDisabledMustBeFirstInValue) {
|
|
FieldTrials f(
|
|
"MyCoolTrial/EnabledFoo/"
|
|
"MyUncoolTrial/DisabledBar/"
|
|
"AnotherTrial/BazEnabled/");
|
|
EXPECT_TRUE(f.IsEnabled("MyCoolTrial"));
|
|
EXPECT_TRUE(f.IsDisabled("MyUncoolTrial"));
|
|
EXPECT_FALSE(f.IsEnabled("AnotherTrial"));
|
|
}
|
|
|
|
TEST(FieldTrialsTest, FieldTrialsDoesNotReadGlobalString) {
|
|
static constexpr char s[] = "MyCoolTrial/Enabled/MyUncoolTrial/Disabled/";
|
|
webrtc::field_trial::InitFieldTrialsFromString(s);
|
|
FieldTrials f("");
|
|
EXPECT_FALSE(f.IsEnabled("MyCoolTrial"));
|
|
EXPECT_FALSE(f.IsDisabled("MyUncoolTrial"));
|
|
}
|
|
|
|
TEST(FieldTrialsTest, FieldTrialsWritesGlobalString) {
|
|
FieldTrials f("MyCoolTrial/Enabled/MyUncoolTrial/Disabled/");
|
|
EXPECT_TRUE(webrtc::field_trial::IsEnabled("MyCoolTrial"));
|
|
EXPECT_TRUE(webrtc::field_trial::IsDisabled("MyUncoolTrial"));
|
|
}
|
|
|
|
TEST(FieldTrialsTest, FieldTrialsRestoresGlobalStringAfterDestruction) {
|
|
static constexpr char s[] = "SomeString/Enabled/";
|
|
webrtc::field_trial::InitFieldTrialsFromString(s);
|
|
{
|
|
FieldTrials f("SomeOtherString/Enabled/");
|
|
EXPECT_STREQ(webrtc::field_trial::GetFieldTrialString(),
|
|
"SomeOtherString/Enabled/");
|
|
}
|
|
EXPECT_STREQ(webrtc::field_trial::GetFieldTrialString(), s);
|
|
}
|
|
|
|
#if GTEST_HAS_DEATH_TEST && !defined(WEBRTC_ANDROID)
|
|
TEST(FieldTrialsTest, FieldTrialsDoesNotSupportSimultaneousInstances) {
|
|
FieldTrials f("SomeString/Enabled/");
|
|
RTC_EXPECT_DEATH(FieldTrials("SomeOtherString/Enabled/").Lookup("Whatever"),
|
|
"Only one instance");
|
|
}
|
|
#endif // GTEST_HAS_DEATH_TEST && !defined(WEBRTC_ANDROID)
|
|
|
|
TEST(FieldTrialsTest, FieldTrialsSupportsSeparateInstances) {
|
|
{ FieldTrials f("SomeString/Enabled/"); }
|
|
{ FieldTrials f("SomeOtherString/Enabled/"); }
|
|
}
|
|
|
|
TEST(FieldTrialsTest, NonGlobalFieldTrialsInstanceDoesNotModifyGlobalString) {
|
|
std::unique_ptr<FieldTrials> f =
|
|
FieldTrials::CreateNoGlobal("SomeString/Enabled/");
|
|
ASSERT_THAT(f, NotNull());
|
|
EXPECT_TRUE(f->IsEnabled("SomeString"));
|
|
EXPECT_FALSE(webrtc::field_trial::IsEnabled("SomeString"));
|
|
}
|
|
|
|
TEST(FieldTrialsTest, NonGlobalFieldTrialsSupportSimultaneousInstances) {
|
|
std::unique_ptr<FieldTrials> f1 =
|
|
FieldTrials::CreateNoGlobal("SomeString/Enabled/");
|
|
std::unique_ptr<FieldTrials> f2 =
|
|
FieldTrials::CreateNoGlobal("SomeOtherString/Enabled/");
|
|
ASSERT_THAT(f1, NotNull());
|
|
ASSERT_THAT(f2, NotNull());
|
|
|
|
EXPECT_TRUE(f1->IsEnabled("SomeString"));
|
|
EXPECT_FALSE(f1->IsEnabled("SomeOtherString"));
|
|
|
|
EXPECT_FALSE(f2->IsEnabled("SomeString"));
|
|
EXPECT_TRUE(f2->IsEnabled("SomeOtherString"));
|
|
}
|
|
|
|
TEST(FieldTrialsTest, GlobalAndNonGlobalFieldTrialsAreDisjoint) {
|
|
FieldTrials f1("SomeString/Enabled/");
|
|
std::unique_ptr<FieldTrials> f2 =
|
|
FieldTrials::CreateNoGlobal("SomeOtherString/Enabled/");
|
|
ASSERT_THAT(f2, NotNull());
|
|
|
|
EXPECT_TRUE(f1.IsEnabled("SomeString"));
|
|
EXPECT_FALSE(f1.IsEnabled("SomeOtherString"));
|
|
|
|
EXPECT_FALSE(f2->IsEnabled("SomeString"));
|
|
EXPECT_TRUE(f2->IsEnabled("SomeOtherString"));
|
|
}
|
|
|
|
TEST(FieldTrialsTest, FieldTrialBasedConfigReadsGlobalString) {
|
|
static constexpr char s[] = "MyCoolTrial/Enabled/MyUncoolTrial/Disabled/";
|
|
webrtc::field_trial::InitFieldTrialsFromString(s);
|
|
FieldTrialBasedConfig f;
|
|
EXPECT_TRUE(f.IsEnabled("MyCoolTrial"));
|
|
EXPECT_TRUE(f.IsDisabled("MyUncoolTrial"));
|
|
}
|
|
|
|
} // namespace
|
|
} // namespace webrtc
|