mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
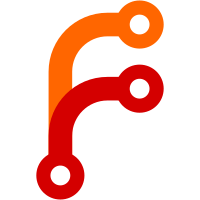
This patch adds new enum values for different types of cellular connections. The new costs are currently blocked when sending to remote, (so that arbitrary network switches does not starts occurring). The end-game for this series to be able to distinguish between different type of cellular connections in the ice-layer (e.g when selecting/switching connections). BUG: webrtc:11473 Change-Id: I587ac8fdff4f6cdd0f8905f327232f58818db4f6 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/172582 Commit-Queue: Jonas Oreland <jonaso@webrtc.org> Reviewed-by: Harald Alvestrand <hta@webrtc.org> Cr-Commit-Position: refs/heads/master@{#30970}
57 lines
1.9 KiB
C++
57 lines
1.9 KiB
C++
/*
|
|
* Copyright 2004 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef RTC_BASE_NETWORK_CONSTANTS_H_
|
|
#define RTC_BASE_NETWORK_CONSTANTS_H_
|
|
|
|
#include <stdint.h>
|
|
|
|
#include <string>
|
|
|
|
namespace rtc {
|
|
|
|
constexpr uint16_t kNetworkCostMax = 999;
|
|
constexpr uint16_t kNetworkCostCellular2G = 980;
|
|
constexpr uint16_t kNetworkCostCellular3G = 910;
|
|
constexpr uint16_t kNetworkCostCellular = 900;
|
|
constexpr uint16_t kNetworkCostCellular4G = 500;
|
|
constexpr uint16_t kNetworkCostCellular5G = 250;
|
|
constexpr uint16_t kNetworkCostUnknown = 50;
|
|
constexpr uint16_t kNetworkCostLow = 10;
|
|
constexpr uint16_t kNetworkCostMin = 0;
|
|
|
|
// alias
|
|
constexpr uint16_t kNetworkCostHigh = kNetworkCostCellular;
|
|
|
|
enum AdapterType {
|
|
// This enum resembles the one in Chromium net::ConnectionType.
|
|
ADAPTER_TYPE_UNKNOWN = 0,
|
|
ADAPTER_TYPE_ETHERNET = 1 << 0,
|
|
ADAPTER_TYPE_WIFI = 1 << 1,
|
|
ADAPTER_TYPE_CELLULAR = 1 << 2, // This is CELLULAR of unknown type.
|
|
ADAPTER_TYPE_VPN = 1 << 3,
|
|
ADAPTER_TYPE_LOOPBACK = 1 << 4,
|
|
// ADAPTER_TYPE_ANY is used for a network, which only contains a single "any
|
|
// address" IP address (INADDR_ANY for IPv4 or in6addr_any for IPv6), and can
|
|
// use any/all network interfaces. Whereas ADAPTER_TYPE_UNKNOWN is used
|
|
// when the network uses a specific interface/IP, but its interface type can
|
|
// not be determined or not fit in this enum.
|
|
ADAPTER_TYPE_ANY = 1 << 5,
|
|
ADAPTER_TYPE_CELLULAR_2G = 1 << 6,
|
|
ADAPTER_TYPE_CELLULAR_3G = 1 << 7,
|
|
ADAPTER_TYPE_CELLULAR_4G = 1 << 8,
|
|
ADAPTER_TYPE_CELLULAR_5G = 1 << 9
|
|
};
|
|
|
|
std::string AdapterTypeToString(AdapterType type);
|
|
|
|
} // namespace rtc
|
|
|
|
#endif // RTC_BASE_NETWORK_CONSTANTS_H_
|