mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
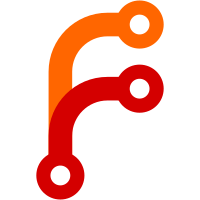
Now that we have moved WebRTC from src/webrtc to src/, common_types.h and typedefs.h are triggering a cpplint error. The cpplint complaint is: Include the directory when naming .h files [build/include] [4] This CL disables the error but we have to remove these two headers from the root directory. NOPRESUBMIT=true Bug: webrtc:5876 Change-Id: I08e1b69aadcc4b28ab83bf25e3819d135d41d333 Reviewed-on: https://webrtc-review.googlesource.com/1577 Commit-Queue: Mirko Bonadei <mbonadei@webrtc.org> Reviewed-by: Henrik Kjellander <kjellander@google.com> Reviewed-by: Karl Wiberg <kwiberg@webrtc.org> Cr-Commit-Position: refs/heads/master@{#19859}
50 lines
1.6 KiB
C++
50 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2014 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_AUDIO_CODING_NETEQ_TOOLS_PACKET_SOURCE_H_
|
|
#define MODULES_AUDIO_CODING_NETEQ_TOOLS_PACKET_SOURCE_H_
|
|
|
|
#include <bitset>
|
|
#include <memory>
|
|
|
|
#include "modules/audio_coding/neteq/tools/packet.h"
|
|
#include "rtc_base/constructormagic.h"
|
|
#include "typedefs.h" // NOLINT(build/include)
|
|
|
|
namespace webrtc {
|
|
namespace test {
|
|
|
|
// Interface class for an object delivering RTP packets to test applications.
|
|
class PacketSource {
|
|
public:
|
|
PacketSource();
|
|
virtual ~PacketSource();
|
|
|
|
// Returns next packet. Returns nullptr if the source is depleted, or if an
|
|
// error occurred.
|
|
virtual std::unique_ptr<Packet> NextPacket() = 0;
|
|
|
|
virtual void FilterOutPayloadType(uint8_t payload_type);
|
|
|
|
virtual void SelectSsrc(uint32_t ssrc);
|
|
|
|
protected:
|
|
std::bitset<128> filter_; // Payload type is 7 bits in the RFC.
|
|
// If SSRC filtering discards all packet that do not match the SSRC.
|
|
bool use_ssrc_filter_; // True when SSRC filtering is active.
|
|
uint32_t ssrc_; // The selected SSRC. All other SSRCs will be discarded.
|
|
|
|
private:
|
|
RTC_DISALLOW_COPY_AND_ASSIGN(PacketSource);
|
|
};
|
|
|
|
} // namespace test
|
|
} // namespace webrtc
|
|
#endif // MODULES_AUDIO_CODING_NETEQ_TOOLS_PACKET_SOURCE_H_
|