mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-12 21:30:45 +01:00
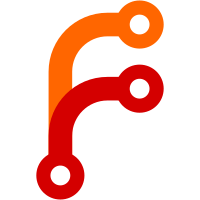
Blink RTC video encoder will need this simplified parser to replace current full H.264 SPS parser, to reduce parsing cost for extracting frame size from compressed H.264 bitstream. Bug: b:309132190 Change-Id: I6863f10bd139766d47fe4bff89143c1a91a09287 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/332468 Reviewed-by: Harald Alvestrand <hta@webrtc.org> Commit-Queue: Jianlin Qiu <jianlin.qiu@intel.com> Cr-Commit-Position: refs/heads/main@{#41466}
53 lines
1.8 KiB
C++
53 lines
1.8 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef COMMON_VIDEO_H264_SPS_PARSER_H_
|
|
#define COMMON_VIDEO_H264_SPS_PARSER_H_
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "rtc_base/bitstream_reader.h"
|
|
#include "rtc_base/system/rtc_export.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// A class for parsing out sequence parameter set (SPS) data from an H264 NALU.
|
|
class RTC_EXPORT SpsParser {
|
|
public:
|
|
// The parsed state of the SPS. Only some select values are stored.
|
|
// Add more as they are actually needed.
|
|
struct RTC_EXPORT SpsState {
|
|
SpsState();
|
|
SpsState(const SpsState&);
|
|
~SpsState();
|
|
|
|
uint32_t width = 0;
|
|
uint32_t height = 0;
|
|
uint32_t delta_pic_order_always_zero_flag = 0;
|
|
uint32_t separate_colour_plane_flag = 0;
|
|
uint32_t frame_mbs_only_flag = 0;
|
|
uint32_t log2_max_frame_num = 4; // Smallest valid value.
|
|
uint32_t log2_max_pic_order_cnt_lsb = 4; // Smallest valid value.
|
|
uint32_t pic_order_cnt_type = 0;
|
|
uint32_t max_num_ref_frames = 0;
|
|
uint32_t vui_params_present = 0;
|
|
uint32_t id = 0;
|
|
};
|
|
|
|
// Unpack RBSP and parse SPS state from the supplied buffer.
|
|
static absl::optional<SpsState> ParseSps(const uint8_t* data, size_t length);
|
|
|
|
protected:
|
|
// Parse the SPS state, up till the VUI part, for a buffer where RBSP
|
|
// decoding has already been performed.
|
|
static absl::optional<SpsState> ParseSpsUpToVui(BitstreamReader& reader);
|
|
};
|
|
|
|
} // namespace webrtc
|
|
#endif // COMMON_VIDEO_H264_SPS_PARSER_H_
|