mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 06:10:40 +01:00
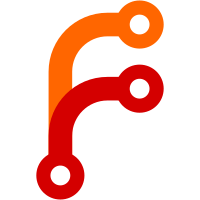
This CL introduces two related changes 1) It changes the way that the AEC3 determines whether the linear filter is sufficiently good for its output to be used. The new scheme achieves this much earlier than what was done in the legacy scheme. 2) It changes the way that saturated echo is and handled so that the impact of the nearend speech is lower. Bug: webrtc:9835,webrtc:9843,chromium:895435,chromium:895431 Change-Id: I0b493676886e2134205e9992bbe4badac7e414cc Reviewed-on: https://webrtc-review.googlesource.com/c/104380 Commit-Queue: Per Åhgren <peah@webrtc.org> Reviewed-by: Gustaf Ullberg <gustaf@webrtc.org> Cr-Commit-Position: refs/heads/master@{#25208}
55 lines
1.8 KiB
C++
55 lines
1.8 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "modules/audio_processing/aec3/subtractor_output.h"
|
|
|
|
#include <numeric>
|
|
|
|
namespace webrtc {
|
|
|
|
SubtractorOutput::SubtractorOutput() = default;
|
|
SubtractorOutput::~SubtractorOutput() = default;
|
|
|
|
void SubtractorOutput::Reset() {
|
|
s_main.fill(0.f);
|
|
s_shadow.fill(0.f);
|
|
e_main.fill(0.f);
|
|
e_shadow.fill(0.f);
|
|
E_main.re.fill(0.f);
|
|
E_main.im.fill(0.f);
|
|
E2_main.fill(0.f);
|
|
E2_shadow.fill(0.f);
|
|
e2_main = 0.f;
|
|
e2_shadow = 0.f;
|
|
s2_main = 0.f;
|
|
s2_shadow = 0.f;
|
|
y2 = 0.f;
|
|
}
|
|
|
|
void SubtractorOutput::ComputeMetrics(rtc::ArrayView<const float> y) {
|
|
const auto sum_of_squares = [](float a, float b) { return a + b * b; };
|
|
y2 = std::accumulate(y.begin(), y.end(), 0.f, sum_of_squares);
|
|
e2_main = std::accumulate(e_main.begin(), e_main.end(), 0.f, sum_of_squares);
|
|
e2_shadow =
|
|
std::accumulate(e_shadow.begin(), e_shadow.end(), 0.f, sum_of_squares);
|
|
s2_main = std::accumulate(s_main.begin(), s_main.end(), 0.f, sum_of_squares);
|
|
s2_shadow =
|
|
std::accumulate(s_shadow.begin(), s_shadow.end(), 0.f, sum_of_squares);
|
|
|
|
s_main_max_abs = *std::max_element(s_main.begin(), s_main.end());
|
|
s_main_max_abs = std::max(s_main_max_abs,
|
|
-(*std::min_element(s_main.begin(), s_main.end())));
|
|
|
|
s_shadow_max_abs = *std::max_element(s_shadow.begin(), s_shadow.end());
|
|
s_shadow_max_abs = std::max(
|
|
s_shadow_max_abs, -(*std::min_element(s_shadow.begin(), s_shadow.end())));
|
|
}
|
|
|
|
} // namespace webrtc
|