mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 22:30:40 +01:00
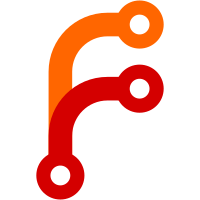
The RWLockWin::Create() function returns NULL on some Windows platforms because it cannot load kernel32.dll. This causes a crash. RWLockWin tries to load kernel32.dll to check if the Slim Reader/Writer Lock APIs are present in kernel32.dll but on newer Windows platforms, kernel32.dll does not exist and the APIs are exported by kernelbase.dll instead. The fix is quite simple: There is no need to try to load any DLL to check if the Slim Reader/Writer Lock APIs are present, because these APIs are always present in all Windows versions since Windows Vista. I am removing the code that attempts to load kernel32.dll. This prevents the crash on platforms that use kernelbase.dll. If the WINUWP preprocessor symbol is defined, RWLockWin was already doing the right thing. But this issue is not limited to WINUWP and in some scenarios, building for WINUWP is not the right solution because it causes other problems. So, my fix is essentially to use the WINUWP code path for all Windows builds. The only version of Windows which does not have the Slim Reader/Writer Lock APIs is Windows XP (and older ones, of course.) However, since the current code does not fall back to an alternative implementation when the Slim Reader/Writer Lock APIs are missing, WebRTC is already broken on such old versions of Windows. Bug: webrtc:11186 Change-Id: I34aad066e18b924792d47c244ecee00669e86c4d Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/161472 Commit-Queue: Tommi <tommi@webrtc.org> Reviewed-by: Karl Wiberg <kwiberg@webrtc.org> Reviewed-by: Henrik Andreassson <henrika@webrtc.org> Reviewed-by: Tommi <tommi@webrtc.org> Cr-Commit-Position: refs/heads/master@{#30044}
41 lines
962 B
C++
41 lines
962 B
C++
/*
|
|
* Copyright (c) 2012 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "rtc_base/synchronization/rw_lock_win.h"
|
|
|
|
#include "rtc_base/logging.h"
|
|
|
|
namespace webrtc {
|
|
|
|
RWLockWin::RWLockWin() {
|
|
InitializeSRWLock(&lock_);
|
|
}
|
|
|
|
RWLockWin* RWLockWin::Create() {
|
|
return new RWLockWin();
|
|
}
|
|
|
|
void RWLockWin::AcquireLockExclusive() {
|
|
AcquireSRWLockExclusive(&lock_);
|
|
}
|
|
|
|
void RWLockWin::ReleaseLockExclusive() {
|
|
ReleaseSRWLockExclusive(&lock_);
|
|
}
|
|
|
|
void RWLockWin::AcquireLockShared() {
|
|
AcquireSRWLockShared(&lock_);
|
|
}
|
|
|
|
void RWLockWin::ReleaseLockShared() {
|
|
ReleaseSRWLockShared(&lock_);
|
|
}
|
|
|
|
} // namespace webrtc
|