mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 14:20:45 +01:00
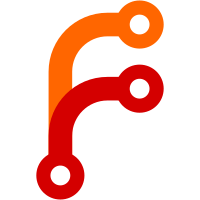
Flakiness of the test reveals this assumption doesn't hold and shouldn't be rely on. Currently there is no code that use it. Plans to rely on it silently adjusted. Bug: webrtc:8610 Change-Id: Id24f2a36c8fb188b518f5301c4b278836885d140 Reviewed-on: https://webrtc-review.googlesource.com/56860 Reviewed-by: Niels Moller <nisse@webrtc.org> Commit-Queue: Danil Chapovalov <danilchap@webrtc.org> Cr-Commit-Position: refs/heads/master@{#22160}
55 lines
2.1 KiB
C++
55 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2015 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_RTP_RTCP_SOURCE_TIME_UTIL_H_
|
|
#define MODULES_RTP_RTCP_SOURCE_TIME_UTIL_H_
|
|
|
|
#include <stdint.h>
|
|
|
|
#include "system_wrappers/include/ntp_time.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// Converts time obtained using rtc::TimeMicros to ntp format.
|
|
// TimeMicrosToNtp guarantees difference of the returned values matches
|
|
// difference of the passed values.
|
|
// As a result TimeMicrosToNtp(rtc::TimeMicros()) doesn't guarantee to match
|
|
// system time.
|
|
NtpTime TimeMicrosToNtp(int64_t time_us);
|
|
|
|
// Converts NTP timestamp to RTP timestamp.
|
|
inline uint32_t NtpToRtp(NtpTime ntp, uint32_t freq) {
|
|
uint32_t tmp = (static_cast<uint64_t>(ntp.fractions()) * freq) >> 32;
|
|
return ntp.seconds() * freq + tmp;
|
|
}
|
|
|
|
// Helper function for compact ntp representation:
|
|
// RFC 3550, Section 4. Time Format.
|
|
// Wallclock time is represented using the timestamp format of
|
|
// the Network Time Protocol (NTP).
|
|
// ...
|
|
// In some fields where a more compact representation is
|
|
// appropriate, only the middle 32 bits are used; that is, the low 16
|
|
// bits of the integer part and the high 16 bits of the fractional part.
|
|
inline uint32_t CompactNtp(NtpTime ntp) {
|
|
return (ntp.seconds() << 16) | (ntp.fractions() >> 16);
|
|
}
|
|
|
|
// Converts interval in microseconds to compact ntp (1/2^16 seconds) resolution.
|
|
// Negative values converted to 0, Overlarge values converted to max uint32_t.
|
|
uint32_t SaturatedUsToCompactNtp(int64_t us);
|
|
|
|
// Converts interval between compact ntp timestamps to milliseconds.
|
|
// This interval can be up to ~9.1 hours (2^15 seconds).
|
|
// Values close to 2^16 seconds consider negative and result in minimum rtt = 1.
|
|
int64_t CompactNtpRttToMs(uint32_t compact_ntp_interval);
|
|
|
|
} // namespace webrtc
|
|
#endif // MODULES_RTP_RTCP_SOURCE_TIME_UTIL_H_
|