mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 06:10:40 +01:00
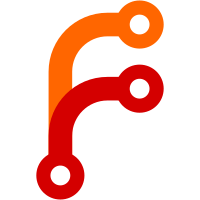
This reverts commit8bf3210629
. Reason for revert: Initialized an uninitialized member in GofInfoVP9 (+ removed some redundant initialization of members already initialized by SetGofInfoVP9()) Original change's description: > Revert "operator== for VideoFrameMetadata + used in CloneSenderVideoFrame test" > > This reverts commit437bf78ed9
. > > Reason for revert: Breaks upstream project > > Original change's description: > > operator== for VideoFrameMetadata + used in CloneSenderVideoFrame test > > > > Added equality and inequality operators for VideoFrameMetadata and used the equality operator to check that the cloned metadata property is equal to the original metadata in RtpSenderVideoFrameTransformerDelegateTest.CloneSenderVideoFrame. > > > > Also default-initialized VideoFrameMetadata::ssrc_ to 0. > > > > Bug: webrtc:14708 > > Change-Id: If1f5153069bc986061ff9f0a6abaa2a4a5a98dd1 > > Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/293560 > > Commit-Queue: Tove Petersson <tovep@google.com> > > Reviewed-by: Tony Herre <herre@google.com> > > Reviewed-by: Harald Alvestrand <hta@webrtc.org> > > Cr-Commit-Position: refs/heads/main@{#39411} > > Bug: webrtc:14708 > Change-Id: Icbec1b65ed22b89766606cb9514dde6f4e9124be > No-Presubmit: true > No-Tree-Checks: true > No-Try: true > Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/295500 > Bot-Commit: rubber-stamper@appspot.gserviceaccount.com <rubber-stamper@appspot.gserviceaccount.com> > Commit-Queue: Harald Alvestrand <hta@webrtc.org> > Auto-Submit: Andrey Logvin <landrey@webrtc.org> > Reviewed-by: Harald Alvestrand <hta@webrtc.org> > Cr-Commit-Position: refs/heads/main@{#39413} Bug: webrtc:14708 Change-Id: I843d29f7dd0da2c7f16968a7fc08dc02cd359fc1 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/295520 Reviewed-by: Harald Alvestrand <hta@webrtc.org> Reviewed-by: Erik Språng <sprang@webrtc.org> Commit-Queue: Tove Petersson <tovep@google.com> Cr-Commit-Position: refs/heads/main@{#39418}
123 lines
3.6 KiB
C++
123 lines
3.6 KiB
C++
/*
|
|
* Copyright (c) 2023 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "api/video/video_frame_metadata.h"
|
|
|
|
#include "api/video/video_frame.h"
|
|
#include "modules/video_coding/codecs/h264/include/h264_globals.h"
|
|
#include "modules/video_coding/codecs/vp9/include/vp9_globals.h"
|
|
#include "test/gtest.h"
|
|
#include "video/video_receive_stream2.h"
|
|
|
|
namespace webrtc {
|
|
namespace {
|
|
|
|
RTPVideoHeaderH264 ExampleHeaderH264() {
|
|
NaluInfo nalu_info;
|
|
nalu_info.type = 1;
|
|
nalu_info.sps_id = 2;
|
|
nalu_info.pps_id = 3;
|
|
|
|
RTPVideoHeaderH264 header;
|
|
header.nalu_type = 4;
|
|
header.packetization_type = H264PacketizationTypes::kH264StapA;
|
|
header.nalus[0] = nalu_info;
|
|
header.nalus_length = 1;
|
|
header.packetization_mode = H264PacketizationMode::SingleNalUnit;
|
|
return header;
|
|
}
|
|
|
|
RTPVideoHeaderVP9 ExampleHeaderVP9() {
|
|
RTPVideoHeaderVP9 header;
|
|
header.InitRTPVideoHeaderVP9();
|
|
header.inter_pic_predicted = true;
|
|
header.flexible_mode = true;
|
|
header.beginning_of_frame = true;
|
|
header.end_of_frame = true;
|
|
header.ss_data_available = true;
|
|
header.non_ref_for_inter_layer_pred = true;
|
|
header.picture_id = 1;
|
|
header.max_picture_id = 2;
|
|
header.tl0_pic_idx = 3;
|
|
header.temporal_idx = 4;
|
|
header.spatial_idx = 5;
|
|
header.temporal_up_switch = true;
|
|
header.inter_layer_predicted = true;
|
|
header.gof_idx = 6;
|
|
header.num_ref_pics = 1;
|
|
header.pid_diff[0] = 8;
|
|
header.ref_picture_id[0] = 9;
|
|
header.num_spatial_layers = 1;
|
|
header.first_active_layer = 0;
|
|
header.spatial_layer_resolution_present = true;
|
|
header.width[0] = 12;
|
|
header.height[0] = 13;
|
|
header.end_of_picture = true;
|
|
header.gof.SetGofInfoVP9(TemporalStructureMode::kTemporalStructureMode1);
|
|
header.gof.pid_start = 14;
|
|
return header;
|
|
}
|
|
|
|
TEST(VideoFrameMetadataTest, H264MetadataEquality) {
|
|
RTPVideoHeaderH264 header = ExampleHeaderH264();
|
|
|
|
VideoFrameMetadata metadata_lhs;
|
|
metadata_lhs.SetRTPVideoHeaderCodecSpecifics(header);
|
|
|
|
VideoFrameMetadata metadata_rhs;
|
|
metadata_rhs.SetRTPVideoHeaderCodecSpecifics(header);
|
|
|
|
EXPECT_TRUE(metadata_lhs == metadata_rhs);
|
|
EXPECT_FALSE(metadata_lhs != metadata_rhs);
|
|
}
|
|
|
|
TEST(VideoFrameMetadataTest, H264MetadataInequality) {
|
|
RTPVideoHeaderH264 header = ExampleHeaderH264();
|
|
|
|
VideoFrameMetadata metadata_lhs;
|
|
metadata_lhs.SetRTPVideoHeaderCodecSpecifics(header);
|
|
|
|
VideoFrameMetadata metadata_rhs;
|
|
header.nalus[0].type = 17;
|
|
metadata_rhs.SetRTPVideoHeaderCodecSpecifics(header);
|
|
|
|
EXPECT_FALSE(metadata_lhs == metadata_rhs);
|
|
EXPECT_TRUE(metadata_lhs != metadata_rhs);
|
|
}
|
|
|
|
TEST(VideoFrameMetadataTest, VP9MetadataEquality) {
|
|
RTPVideoHeaderVP9 header = ExampleHeaderVP9();
|
|
|
|
VideoFrameMetadata metadata_lhs;
|
|
metadata_lhs.SetRTPVideoHeaderCodecSpecifics(header);
|
|
|
|
VideoFrameMetadata metadata_rhs;
|
|
metadata_rhs.SetRTPVideoHeaderCodecSpecifics(header);
|
|
|
|
EXPECT_TRUE(metadata_lhs == metadata_rhs);
|
|
EXPECT_FALSE(metadata_lhs != metadata_rhs);
|
|
}
|
|
|
|
TEST(VideoFrameMetadataTest, VP9MetadataInequality) {
|
|
RTPVideoHeaderVP9 header = ExampleHeaderVP9();
|
|
|
|
VideoFrameMetadata metadata_lhs;
|
|
metadata_lhs.SetRTPVideoHeaderCodecSpecifics(header);
|
|
|
|
VideoFrameMetadata metadata_rhs;
|
|
header.gof.pid_diff[0][0] = 42;
|
|
metadata_rhs.SetRTPVideoHeaderCodecSpecifics(header);
|
|
|
|
EXPECT_FALSE(metadata_lhs == metadata_rhs);
|
|
EXPECT_TRUE(metadata_lhs != metadata_rhs);
|
|
}
|
|
|
|
} // namespace
|
|
} // namespace webrtc
|