mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 22:30:40 +01:00
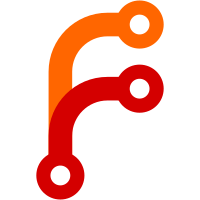
The RtpDemuxer uses a number of maps, all of which can be made unordered as they map SSRCs/MIDs/payload types/RSIDs etc - all of which have no inherent ordering. In busy media servers, the std::map operations can use ~0.5% CPU. After this commit has landed, it will be evaluated and if it doesn't live up to expected savings, it will be reverted. Bug: webrtc:12689 Change-Id: I99e21c6b1ddb21dd9d47b0f9a891df5a2c3df59a Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/216243 Reviewed-by: Sebastian Jansson <srte@webrtc.org> Reviewed-by: Mirko Bonadei <mbonadei@webrtc.org> Commit-Queue: Victor Boivie <boivie@webrtc.org> Cr-Commit-Position: refs/heads/master@{#33846}
49 lines
1.3 KiB
C++
49 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2021 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#include "rtc_base/hash.h"
|
|
|
|
#include <string>
|
|
#include <unordered_map>
|
|
#include <unordered_set>
|
|
|
|
#include "test/gmock.h"
|
|
|
|
namespace webrtc {
|
|
namespace {
|
|
|
|
TEST(PairHashTest, CanInsertIntoSet) {
|
|
using MyPair = std::pair<int, int>;
|
|
|
|
std::unordered_set<MyPair, PairHash> pairs;
|
|
|
|
pairs.insert({1, 2});
|
|
pairs.insert({3, 4});
|
|
|
|
EXPECT_NE(pairs.find({1, 2}), pairs.end());
|
|
EXPECT_NE(pairs.find({3, 4}), pairs.end());
|
|
EXPECT_EQ(pairs.find({1, 3}), pairs.end());
|
|
EXPECT_EQ(pairs.find({3, 3}), pairs.end());
|
|
}
|
|
|
|
TEST(PairHashTest, CanInsertIntoMap) {
|
|
using MyPair = std::pair<std::string, int>;
|
|
|
|
std::unordered_map<MyPair, int, PairHash> pairs;
|
|
|
|
pairs[{"1", 2}] = 99;
|
|
pairs[{"3", 4}] = 100;
|
|
|
|
EXPECT_EQ((pairs[{"1", 2}]), 99);
|
|
EXPECT_EQ((pairs[{"3", 4}]), 100);
|
|
EXPECT_EQ(pairs.find({"1", 3}), pairs.end());
|
|
EXPECT_EQ(pairs.find({"3", 3}), pairs.end());
|
|
}
|
|
} // namespace
|
|
} // namespace webrtc
|