mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 06:10:40 +01:00
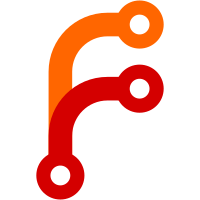
If configured, attempt to negotiate "zero checksum acceptable" capability, which will make the outgoing packets have a fixed checksum of zero. Received packets will not be verified for a correct checksum if it's zero. Also includes some boilerplate: - Setting capability in state cookie - Adding capability to handover state - Adding metric to know if the feature is used This feature is not enabled by default, as it will be first evaluated with an A/B experiment before making it the default. When the feature is enabled, lower CPU consumption for both receiving and sending packets is expected. How much depends on the architecture, as some architectures have better support for generating CRC32 in hardware than others. Bug: webrtc:14997 Change-Id: If23f73e87220c7d42bd4f9a92772cda16bc18fcb Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/299076 Commit-Queue: Victor Boivie <boivie@webrtc.org> Reviewed-by: Florent Castelli <orphis@webrtc.org> Reviewed-by: Harald Alvestrand <hta@webrtc.org> Cr-Commit-Position: refs/heads/main@{#39920}
65 lines
2.1 KiB
C++
65 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2021 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#ifndef NET_DCSCTP_SOCKET_STATE_COOKIE_H_
|
|
#define NET_DCSCTP_SOCKET_STATE_COOKIE_H_
|
|
|
|
#include <cstdint>
|
|
#include <vector>
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "api/array_view.h"
|
|
#include "net/dcsctp/common/internal_types.h"
|
|
#include "net/dcsctp/socket/capabilities.h"
|
|
|
|
namespace dcsctp {
|
|
|
|
// This is serialized as a state cookie and put in INIT_ACK. The client then
|
|
// responds with this in COOKIE_ECHO.
|
|
//
|
|
// NOTE: Expect that the client will modify it to try to exploit the library.
|
|
// Do not trust anything in it; no pointers or anything like that.
|
|
class StateCookie {
|
|
public:
|
|
static constexpr size_t kCookieSize = 37;
|
|
|
|
StateCookie(VerificationTag initiate_tag,
|
|
TSN initial_tsn,
|
|
uint32_t a_rwnd,
|
|
TieTag tie_tag,
|
|
Capabilities capabilities)
|
|
: initiate_tag_(initiate_tag),
|
|
initial_tsn_(initial_tsn),
|
|
a_rwnd_(a_rwnd),
|
|
tie_tag_(tie_tag),
|
|
capabilities_(capabilities) {}
|
|
|
|
// Returns a serialized version of this cookie.
|
|
std::vector<uint8_t> Serialize();
|
|
|
|
// Deserializes the cookie, and returns absl::nullopt if that failed.
|
|
static absl::optional<StateCookie> Deserialize(
|
|
rtc::ArrayView<const uint8_t> cookie);
|
|
|
|
VerificationTag initiate_tag() const { return initiate_tag_; }
|
|
TSN initial_tsn() const { return initial_tsn_; }
|
|
uint32_t a_rwnd() const { return a_rwnd_; }
|
|
TieTag tie_tag() const { return tie_tag_; }
|
|
const Capabilities& capabilities() const { return capabilities_; }
|
|
|
|
private:
|
|
const VerificationTag initiate_tag_;
|
|
const TSN initial_tsn_;
|
|
const uint32_t a_rwnd_;
|
|
const TieTag tie_tag_;
|
|
const Capabilities capabilities_;
|
|
};
|
|
} // namespace dcsctp
|
|
|
|
#endif // NET_DCSCTP_SOCKET_STATE_COOKIE_H_
|