mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
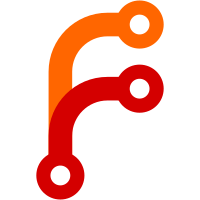
PeerConnection now has a new setting in RTCConfiguration to enable use of datagram transport for data channels. There is also a corresponding field trial, which has both a kill-switch and a way to change the default value. PeerConnection's interaction with MediaTransport for data channels has been refactored to work with DataChannelTransportInterface instead. Adds a DataChannelState and OnStateChanged() to the DataChannelSink callbacks. This allows PeerConnection to listen to the data channel's state directly, instead of indirectly by monitoring media transport state. This is necessary to enable use of non-media-transport (eg. datagram transport) data channel transports. For now, PeerConnection watches the state through MediaTransport as well. This will persist until MediaTransport implements the new callback. Datagram transport use is negotiated. As such, an offer that requests to use datagram transport for data channels may be rejected by the answerer. If the offer includes DTLS, the data channels will be negotiated as SCTP/DTLS data channels with an extra x-opaque parameter for datagram transport. If the opaque parameter is rejected (by an answerer without datagram support), the offerer may fall back to SCTP. If DTLS is not enabled, there is no viable fallback. In this case, the data channels are negotiated as media transport data channels. If the receiver does not understand the x-opaque line, it will reject these data channels, and the offerer's data channels will be closed. Bug: webrtc:9719 Change-Id: Ic1bf3664c4bcf9d754482df59897f5f72fe68fcc Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/147702 Commit-Queue: Bjorn Mellem <mellem@webrtc.org> Reviewed-by: Steve Anton <steveanton@webrtc.org> Cr-Commit-Position: refs/heads/master@{#28932}
40 lines
1.3 KiB
C++
40 lines
1.3 KiB
C++
/* Copyright 2019 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "api/data_channel_transport_interface.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// TODO(mellem): Delete these default implementations and make these functions
|
|
// pure virtual as soon as downstream implementations override them.
|
|
|
|
RTCError DataChannelTransportInterface::OpenChannel(int channel_id) {
|
|
return RTCError(RTCErrorType::UNSUPPORTED_OPERATION);
|
|
}
|
|
|
|
RTCError DataChannelTransportInterface::SendData(
|
|
int channel_id,
|
|
const SendDataParams& params,
|
|
const rtc::CopyOnWriteBuffer& buffer) {
|
|
return RTCError(RTCErrorType::UNSUPPORTED_OPERATION);
|
|
}
|
|
|
|
RTCError DataChannelTransportInterface::CloseChannel(int channel_id) {
|
|
return RTCError(RTCErrorType::UNSUPPORTED_OPERATION);
|
|
}
|
|
|
|
void DataChannelTransportInterface::SetDataSink(DataChannelSink* /*sink*/) {}
|
|
|
|
bool DataChannelTransportInterface::IsReadyToSend() const {
|
|
return false;
|
|
}
|
|
|
|
void DataChannelSink::OnReadyToSend() {}
|
|
|
|
} // namespace webrtc
|