mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
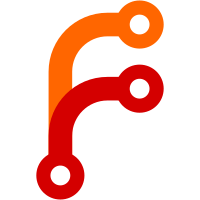
Agc2 applies a digital gain to the nearend signal. When the analog level changes, the digital gain calculation is no longer valid. Therefore Agc2 should be notified to analog gain changes. This CL also allow audioproc_f to chain AGC1 and AGC2. In a dependent CL we will allow using AGC1 for analog gain and AGC2 for digital gain. Bug: webrtc:7494 Change-Id: Id75b3728fbf2de1d84b7fba005e4670c7a2985d9 Reviewed-on: https://webrtc-review.googlesource.com/89387 Commit-Queue: Alex Loiko <aleloi@webrtc.org> Reviewed-by: Alessio Bazzica <alessiob@webrtc.org> Cr-Commit-Position: refs/heads/master@{#24231}
56 lines
1.7 KiB
C++
56 lines
1.7 KiB
C++
/*
|
|
* Copyright (c) 2017 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_AUDIO_PROCESSING_GAIN_CONTROLLER2_H_
|
|
#define MODULES_AUDIO_PROCESSING_GAIN_CONTROLLER2_H_
|
|
|
|
#include <memory>
|
|
#include <string>
|
|
|
|
#include "modules/audio_processing/agc2/adaptive_agc.h"
|
|
#include "modules/audio_processing/agc2/fixed_gain_controller.h"
|
|
#include "modules/audio_processing/include/audio_processing.h"
|
|
#include "rtc_base/constructormagic.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class ApmDataDumper;
|
|
class AudioBuffer;
|
|
|
|
// Gain Controller 2 aims to automatically adjust levels by acting on the
|
|
// microphone gain and/or applying digital gain.
|
|
class GainController2 {
|
|
public:
|
|
GainController2();
|
|
~GainController2();
|
|
|
|
void Initialize(int sample_rate_hz);
|
|
void Process(AudioBuffer* audio);
|
|
void NotifyAnalogLevel(int level);
|
|
|
|
void ApplyConfig(const AudioProcessing::Config::GainController2& config);
|
|
static bool Validate(const AudioProcessing::Config::GainController2& config);
|
|
static std::string ToString(
|
|
const AudioProcessing::Config::GainController2& config);
|
|
|
|
private:
|
|
static int instance_count_;
|
|
std::unique_ptr<ApmDataDumper> data_dumper_;
|
|
FixedGainController fixed_gain_controller_;
|
|
AudioProcessing::Config::GainController2 config_;
|
|
AdaptiveAgc adaptive_agc_;
|
|
int analog_level_ = -1;
|
|
|
|
RTC_DISALLOW_COPY_AND_ASSIGN(GainController2);
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_AUDIO_PROCESSING_GAIN_CONTROLLER2_H_
|